Pointers are a core feature of the C Programming language that allows developers to directly manipulate memory addresses, which can significantly enhance program efficiency and performance. Whether you’re looking to traverse strings, create efficient lookup tables, manage complex tree structures, or streamline data access in control tables, pointers are an essential tool to master. This article dives deep into what pointers are, how they work, and how they can be used in various scenarios.
Table of Contents
What is a Pointer?
A pointer is a variable that stores the address of another variable, not the value itself. This means that, rather than holding the actual data, a pointer holds the location in memory where that data is stored. By using pointers, a program can directly access and modify data in memory, offering greater flexibility and control over how that data is managed.
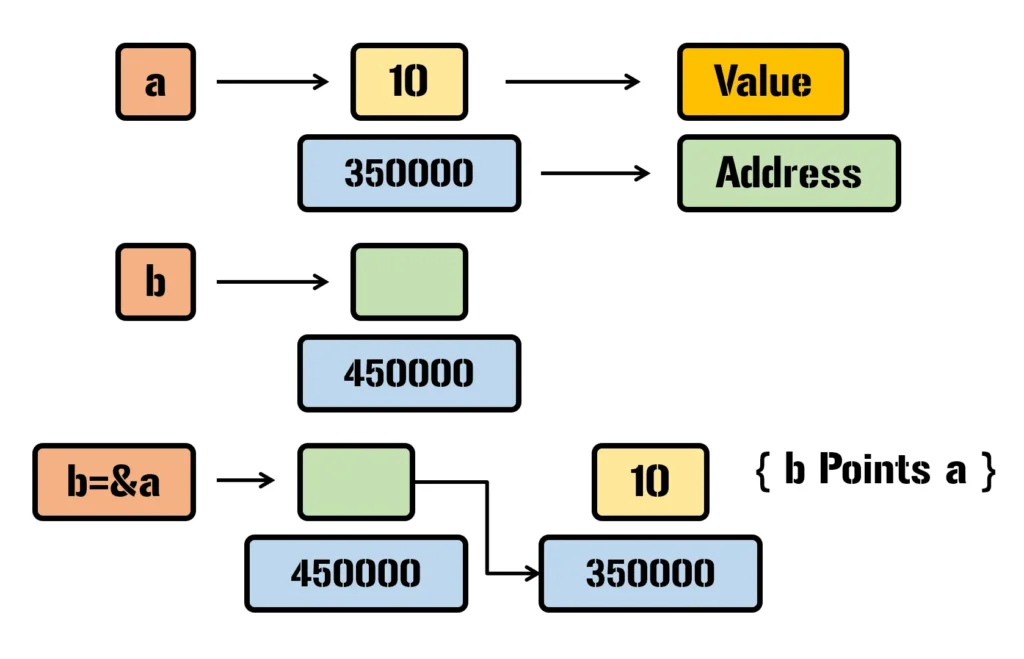
Dereferencing a Pointer
To access or manipulate the value at a given memory address, we use dereferencing. Dereferencing a pointer means using the pointer to retrieve or modify the value stored at the location it points to.
For instance, if a pointer p
holds the address of a variable x
, dereferencing p
gives us the value of x
. Dereferencing is achieved in C by using the *
operator.
Advantages of Using Pointers
- Efficient Memory Management: By accessing and modifying memory directly, pointers reduce the need for redundant copying and improve efficiency, especially in repetitive processes.
- Enhanced Data Structures: Many data structures, like linked lists, trees, and graphs, rely on pointers for their flexibility and dynamic memory management.
- Function Parameters: Passing large data structures by pointer instead of by value allows functions to modify data directly, optimizing both memory usage and processing time.
Pointer Details
To understand pointers more fully, let’s go through various aspects and types of pointers, including pointer arithmetic, arrays of pointers, pointer to pointer, and passing pointers to functions.
Pointer Arithmetic
Pointer arithmetic allows the manipulation of memory addresses using specific operators:
- Increment (
++
): Moves the pointer to the next memory location of its data type. - Decrement (
--
): Moves the pointer to the previous memory location. - Addition (
+
): Adds a specified number to the pointer, moving it that many memory locations forward. - Subtraction (
-
): Moves the pointer backward by a specified number of memory locations.
Example:
int arr[] = {10, 20, 30, 40};
int *p = arr;
p++; // Now p points to arr[1] (20)
Array of Pointers
You can also define an array of pointers in C. This type of array holds multiple memory addresses, making it ideal for handling multiple strings or large arrays of data efficiently.
Example:
const char *names[] = {"Alice", "Bob", "Charlie"};
Each element in names
is a pointer to a string, which allows us to access and modify the strings in various ways.
Pointers in Action: Coding Examples
Let’s take a look at a few sample programs to understand how pointers work in practice, starting with a simple example to demonstrate pointer basics.
Program 1: Basic Pointer Usage
The following program illustrates how pointers store addresses and how to access the value stored at a pointer’s address.
#include <stdio.h>
int main( ) {
int a = 5;
int *b;
b = &a; // Assigning the address of variable a to pointer b
// Outputting values and addresses using pointers
printf ("Value of a = %d\n", a); // Prints 5
printf ("Value of a using *(&a) = %d\n", *(&a)); // Dereferencing &a gives 5
printf ("Value of a using *b = %d\n", *b); // Dereferencing b gives 5
printf ("Address of a = %u\n", &a); // Prints the memory address of a
printf ("Address of b (holding &a) = %u\n", b); // Prints the same address as &a
printf ("Address of pointer b itself = %u\n", &b); // Prints the memory address of b
printf ("Value of b, which is address of a = %u\n", b); // Shows the address of a
return 0;
}
Explanation:
int a = 5;
– Declares an integera
with a value of5
.int *b;
– Declares a pointerb
capable of storing the address of an integer.b = &a;
– Assigns the address ofa
tob
.- Dereferencing
*b
fetches the value at the addressb
is pointing to, which is5
.
Output:
Value of a = 5
Value of a using *(&a) = 5
Value of a using *b = 5
Address of a = 2355636716
Address of b (holding &a) = 2355636716
Address of pointer b itself = 2355636720
Value of b, which is address of a = 2355636716
Program 2: Pointer to Pointer
A pointer to a pointer is a pointer that stores the address of another pointer, creating multiple levels of indirection. This is particularly useful in multidimensional arrays, matrices, and complex data structures.
#include <stdio.h>
int main( ) {
int a = 5;
int *b;
int **c;
b = &a; // Pointer b holds address of a
c = &b; // Pointer c holds address of pointer b
printf ("Value of a = %d\n", a);
printf ("Value of a using *(&a) = %d\n", *(&a));
printf ("Value of a using *b = %d\n", *b);
printf ("Value of a using **c = %d\n", **c); // Dereferencing twice gives value of a
printf ("Value of b (address of a) = %u\n", b);
printf ("Value of c (address of b) = %u\n", c);
printf ("Address of a = %u\n", &a);
printf ("Address of b = %u\n", &b);
printf ("Address of c = %u\n", &c);
return 0;
}
Explanation:
int **c;
– Declares a pointer to a pointer, which can store the address ofb
, which is itself a pointer toa
.**c
– Dereferencesc
twice to reach the value stored ina
.
Output:
Value of a = 5
Value of a using *(&a) = 5
Value of a using *b = 5
Value of a using **c = 5
Value of b (address of a) = 1442298196
Value of c (address of b) = 1442298200
Address of a = 1442298196
Address of b = 1442298200
Address of c = 1442298208
Additional Concepts
Passing Pointers to Functions
In C, passing a pointer to a function instead of a variable enables pass-by-reference. This allows the function to modify the original data, making it more efficient for large data structures.
Example:
void modifyValue(int *p) {
*p = 20;
}
int main() {
int a = 10;
modifyValue(&a);
printf("Modified value of a = %d\n", a); // Outputs 20
return 0;
}
Returning Pointers from Functions
C allows functions to return pointers, though care must be taken with local variables (due to their scope) unless they are declared as static or dynamically allocated.
Conclusion
Pointers are a powerful feature in C programming, enabling developers to work directly with memory addresses, optimize code performance, and create flexible, dynamic data structures. Whether you’re dealing with pointer arithmetic, arrays of pointers, pointers to pointers, or functions involving pointers, understanding these concepts is key to mastering C.
Pointers and Memory Management Across Multiple Programming Languages
Here’s a detailed guide on pointers and memory management across multiple programming languages.
Each example will demonstrate how to create, manipulate, and dereference pointers (or pointer-like references where direct memory manipulation isn’t possible) and describe each line of code for an in-depth understanding.
Code:
#include <stdio.h>
int main() {
int a = 10; // Declares an integer variable a and assigns it the value 10
int *p; // Declares a pointer p to store the address of an integer
p = &a; // Assigns the address of a to pointer p
printf("Value of a = %d\n", a); // Prints the value of a
printf("Value at pointer p = %d\n", *p); // Dereferences pointer p to print the value of a
printf("Address of a = %p\n", (void*)&a); // Prints the memory address of a
printf("Value of pointer p (address of a) = %p\n", (void*)p); // Prints the address stored in p
return 0;
}
Explanation:
int a = 10;
: Declares an integer variablea
with a value of10
.int *p;
: Declares a pointerp
that can store the address of an integer.p = &a;
: Assigns the address ofa
top
, sop
now points toa
.printf("Value of a = %d\n", a);
: Prints the value ofa
, which is10
.printf("Value at pointer p = %d\n", *p);
: Dereferencesp
to get the value stored at the address, printing10
.printf("Address of a = %p\n", (void*)&a);
: Prints the address ofa
in hexadecimal format.
Output:
Value of a = 10
Value at pointer p = 10
Address of a = 0x7ffdf1a7b6c0
Value of pointer p (address of a) = 0x7ffdf1a7b6c0
Code:
#include <iostream>
using namespace std;
int main() {
int a = 20; // Declare an integer variable a with value 20
int *p = &a; // Initialize pointer p with the address of a
cout << "Value of a = " << a << endl; // Outputs value of a
cout << "Value at pointer p = " << *p << endl; // Dereferences p to show value of a
cout << "Address of a = " << &a << endl; // Prints address of a
cout << "Value of pointer p (address of a) = " << p << endl; // Shows address in p
return 0;
}
Explanation:
int *p = &a;
:p
is initialized with the address ofa
, sop
points toa
.
Output:
Value of a = 20
Value at pointer p = 20
Address of a = 0x61ff08
Value of pointer p (address of a) = 0x61ff08
Code:
C# does not directly support pointer arithmetic in standard code, but unsafe code can access pointers if unsafe and fixed keywords are used, along with enabling /unsafe compilation.
using System;
class Program {
unsafe static void Main() {
int a = 30;
int* p = &a;
Console.WriteLine("Value of a = " + a);
Console.WriteLine("Value at pointer p = " + *p);
Console.WriteLine("Address of a = " + (IntPtr)p);
}
}
Explanation:
int* p = &a;
: This line stores the address ofa
in pointerp
. In an unsafe context, pointers can manipulate memory addresses directly.Console.WriteLine("Value at pointer p = " + *p);
: Dereferencesp
to print the value30
.
Output:
Value of a = 30
Value at pointer p = 30
Address of a = 0x000000000063FF08
Code:
Java does not support pointers directly but uses references to manipulate objects. Here’s a reference-based approach.
public class PointerDemo {
public static void main(String[] args) {
Integer a = 40; // Declare and initialize Integer a with value 40
Integer b = a; // Reference b now points to the same Integer object as a
System.out.println("Value of a = " + a); // Outputs the value of a
System.out.println("Value of b (same as a) = " + b); // Outputs the same value as a
System.out.println("Reference comparison (a == b): " + (a == b)); // Checks if a and b reference the same object
}
}
Explanation:
Integer a = 40;
: Initializesa
with40
, which is treated as a reference to an Integer object.
Output:
Value of a = 40
Value of b (same as a) = 40
Reference comparison (a == b): true
Code:
Python handles all variables as references and does not use pointers explicitly. However, we can illustrate reference behavior similar to pointers.
def modify_value(x):
x[0] = 50 # Modifies the first element in the list
a = [30] # Initialize a list with one element
print("Value before modification:", a[0])
modify_value(a) # Passes a reference to the list
print("Value after modification:", a[0])
Explanation:
a = [30];
: Initializesa
as a list with one element30
. Lists are mutable and passed by reference.modify_value(a);
: Passes the lista
tomodify_value
, which modifies the first element ofa
to50
.
Output:
Value before modification: 30
Value after modification: 50
Comparison of Pointer Behavior Across Languages
- C and C++: Allow direct pointer manipulation and arithmetic, essential for system programming.
- C#: Has limited pointer functionality in unsafe blocks.
- Java: Uses references and lacks explicit pointer manipulation.
- Python: Uses references and immutable/mutable types to manage data manipulation rather than pointers.
These examples provide insights into pointer usage and behavior across languages and can be especially useful for understanding memory management across various programming paradigms.
Read More Articles
- Data Structure (DS) Array:
- Why the Analysis of Algorithms is Important?
- Worst, Average, and Best Case Analysis of Algorithms: A Comprehensive Guide
- Understanding Pointers in C Programming: A Comprehensive Guide
- Understanding Arrays in Data Structures: A Comprehensive Exploration
- Memory Allocation of an Array: An In-Depth Comprehensive Exploration
- Understanding Basic Operations in Arrays: A Comprehensive Guide
- Understanding 2D Arrays in Programming: A Comprehensive Guide
- Mapping a 2D Array to a 1D Array: A Comprehensive Exploration
- Data Structure Linked List:
- Understanding Linked Lists in Data Structures: A Comprehensive Exploration
- Types of Linked List: Detailed Exploration, Representations, and Implementations
- Understanding Singly Linked Lists: A Detailed Exploration
- Understanding Doubly Linked List: A Comprehensive Guide
- Operations of Doubly Linked List with Implementation: A Detailed Exploration
- Insertion in Doubly Linked List with Implementation: A Detailed Exploration
- Inserting a Node at the beginning of a Doubly Linked List: A Detailed Exploration
- Inserting a Node After a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Inserting a New Node at the End of a Doubly Linked List: A Detailed Exploration
- Deletion in a Doubly Linked List with Implementation: A Comprehensive Guide
- Deletion at the Beginning in a Doubly Linked List: A Detailed Exploration
- Deletion after a given node in Doubly Linked List: A Comprehensive Guide
- Deleting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Deletion at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Deletion at the End in Doubly Linked List: A Comprehensive Exploration
- Introduction to Circular Linked Lists: A Comprehensive Guide
- Understanding Circular Singly Linked Lists: A Comprehensive Guide
- Circular Doubly Linked List: A Comprehensive Guide
- Insertion in Circular Singly Linked List: A Comprehensive Guide
- Insertion in an Empty Circular Linked List: A Detailed Exploration
- Insertion at the Beginning in Circular Linked List: A Detailed Exploration
- Insertion at the End of a Circular Linked List: A Comprehensive Guide
- Insertion at a Specific Position in a Circular Linked List: A Detailed Exploration
- Deletion from a Circular Linked List: A Comprehensive Guide
- Deletion from the Beginning of a Circular Linked List: A Detailed Exploration
- Deletion at Specific Position in Circular Linked List: A Detailed Exploration
- Deletion at the End of a Circular Linked List: A Comprehensive Guide
- Searching in a Circular Linked List: A Comprehensive Exploration
Frequently Asked Questions (FAQs)
What is a pointer, and how does it work in programming?
A pointer is a variable that stores the address of another variable rather than storing a direct value. In languages like C and C++, pointers enable direct memory access and manipulation, making operations faster and more memory-efficient. Instead of storing an integer or a character, a pointer variable holds the memory address where that data resides.
For example, consider the following in C:
int a = 10;
int *p = &a;
Here, a
holds the integer value 10
, while p
holds the memory address of a
. Dereferencing the pointer, *p
, gives access to the actual value of a
(which is 10
). This method enables programs to manipulate large datasets by reference instead of copying, enhancing efficiency in complex operations.
What are the main uses of pointers in programming?
Pointers have several applications, especially in low-level programming languages. Here are some primary uses:
- Dynamic Memory Allocation: In C and C++, pointers allow allocation and deallocation of memory at runtime using functions like
malloc()
,calloc()
, andfree()
. This is essential for managing memory in programs with large or variable datasets. - Data Structure Management: Pointers are crucial for implementing linked lists, trees, and graphs. Each element in a linked list, for instance, contains a pointer to the next element, enabling efficient insertion and deletion.
- Pass-by-Reference in Functions: Pointers allow pass-by-reference, enabling functions to modify the original data rather than a copy, saving memory and improving performance.
- System-Level Programming: In systems programming, pointers give access to hardware, memory-mapped registers, and efficient data manipulation at a low level.
What is pointer arithmetic, and why is it useful?
Pointer arithmetic is the ability to manipulate memory addresses stored in pointers. This feature is highly useful in C and C++ for navigating arrays and data structures. Only four operations are allowed: ++
, --
, +
, and -
.
Example:
int arr[] = {10, 20, 30};
int *p = arr;
p++; // Now p points to arr[1], which is 20
Here, incrementing p
moves it to the next integer’s address. This type of arithmetic is efficient because it lets developers traverse arrays and other structures without needing array indices or additional variables. Pointer arithmetic is a key reason why C/C++ are considered efficient for operations requiring rapid data access, such as graphics or systems programming.
How do pointers differ in C, C++, C#, Java, and Python?
Pointers have varying degrees of accessibility across languages:
- C and C++: Support explicit pointer manipulation, including arithmetic. C is closer to hardware, while C++ supports pointers with added safety features like smart pointers.
- C#: Limited pointer support is available within unsafe blocks. This is generally discouraged in high-level code but can be used in performance-sensitive sections.
- Java: Does not support pointers but uses references for object manipulation. Java’s Garbage Collector (GC) handles memory management automatically.
- Python: No direct pointer support; it uses references to manage objects, and memory management is handled through an automatic garbage collector.
Each language balances memory safety with performance in different ways, reflecting its design philosophy and typical application areas.
What is the difference between NULL
and an uninitialized pointer?
In C and C++, NULL
represents a pointer that points to no valid memory address. An uninitialized pointer, on the other hand, contains a random memory address.
Example:
int *ptr1 = NULL; // This pointer is explicitly set to NULL
int *ptr2; // This pointer is uninitialized and may point to an unknown address
Dereferencing an uninitialized pointer may lead to undefined behavior, such as accessing or modifying unintended memory locations. By contrast, dereferencing NULL
typically results in a segmentation fault (a runtime error). Using NULL
helps indicate that a pointer isn’t pointing to valid data, aiding in debugging and reducing potential errors.
What is a “pointer to a pointer,” and when would you use one?
A pointer to a pointer is a variable that stores the address of another pointer. This enables multi-level indirection, which can be helpful in complex data structures and dynamic memory allocation.
Example in C:
int a = 5;
int *p1 = &a; // Pointer to int
int **p2 = &p1; // Pointer to pointer to int
Pointer to pointer usage is common when working with arrays of pointers, multi-dimensional arrays, and dynamic structures (such as managing linked list heads). They also facilitate passing references to dynamically allocated memory when dealing with complex nested data structures.
What is dereferencing a pointer, and how does it work?
Dereferencing a pointer means accessing the value stored at the memory address the pointer holds. In C and C++, this is done using the *
operator. Dereferencing is essential for working with pointer data, as it allows a program to read or modify the original data instead of a copy.
Example:
int a = 10;
int *p = &a;
int value = *p; // Dereferences p to retrieve the value of a, which is 10
Dereferencing enables efficient data manipulation by working directly with memory locations. However, if dereferencing a pointer that is NULL
or uninitialized, it can cause crashes or unexpected behavior.
How do you pass pointers to functions, and what are the benefits?
Passing pointers to functions, known as pass-by-reference, allows functions to modify the original data rather than a copy. This improves memory usage and performance, especially when dealing with large data structures.
Example in C:
void modify(int *p) {
*p = 20;
}
int main() {
int a = 10;
modify(&a); // Passing address of a
printf("%d", a); // Outputs 20, since a was modified
return 0;
}
Here, the function modify
takes a pointer as an argument, enabling it to change the value of a
directly. This is faster than copying large amounts of data, especially in applications involving complex data structures.
What is dynamic memory allocation, and how is it used with pointers?
Dynamic memory allocation is the process of allocating memory at runtime, rather than at compile time. In C and C++, functions like malloc()
, calloc()
, realloc()
, and free()
enable dynamic memory management.
Example in C:
int *p = (int*)malloc(sizeof(int) * 5); // Allocates memory for an array of 5 integers
p[0] = 10; // Assigns value to dynamically allocated memory
free(p); // Frees the allocated memory
Dynamic memory allocation allows programs to handle variable-sized data, such as user input, files, or network data, more flexibly. After usage, deallocation is crucial to prevent memory leaks, which can cause applications to consume more memory over time.
What are smart pointers in C++, and why are they used?
Smart pointers are an enhancement in C++ that manages memory automatically. Introduced in C++11 with <memory>
library, smart pointers include classes like std::unique_ptr
, std::shared_ptr
, and std::weak_ptr
. They ensure that dynamically allocated memory is automatically freed, reducing memory leaks and manual memory management.
Example of std::unique_ptr
:
#include <memory>
#include <iostream>
int main() {
std::unique_ptr<int> ptr(new int(10)); // Creates a unique_ptr managing an int
std::cout << "Value: " << *ptr << std::endl;
return 0; // Memory automatically freed when ptr goes out of scope
}
In the example, std::unique_ptr
takes ownership of the int
, and when ptr
goes out of scope, the memory is freed automatically. Smart pointers provide a safer, more modern way to manage memory, reducing issues like double frees, dangling pointers, and memory leaks often encountered in manual memory management.