Linked lists are a fundamental data structure in computer science, offering dynamic memory management and flexibility in data manipulation. This article explores the various types of linked lists, their structures, use cases, and differences. Let’s dive into the details to build a robust understanding of this topic.
Table of Contents
What is a Linked List?
A linked list is a linear data structure where each element, called a node, contains two parts:
- Data Part: Holds the actual value or information.
- Pointer Part: Contains the address of the next node in the sequence.
Unlike arrays, linked lists do not require contiguous memory allocation, making them highly efficient for dynamic memory applications.
Types of Linked Lists
Types of Linked Lists can be broadly categorized into four main types, each designed to suit specific use cases.
- A Singly Linked List is the simplest form, where each node has a data part and a pointer to the next node, allowing only forward traversal.
- A Doubly Linked List extends this by adding a pointer to the previous node, enabling bidirectional traversal.
- In a Circular Linked List, the last node points back to the first, forming a continuous loop and supporting cyclic operations, typically in forward direction.
- Finally, a Doubly Circular Linked List combines features of both doubly and circular lists, where nodes have pointers to both previous and next nodes, and the last node connects back to the first, enabling seamless traversal in both directions.
Each type has distinct advantages for various applications in data structures and algorithms.
1. Singly Linked List
A singly linked list is the most commonly used type of linked list. Each node in this list contains:
- Data: The value to be stored.
- Next Pointer: A pointer to the next node in the sequence.
Characteristics
- Forward Traversal Only: Traversal is unidirectional, starting from the head node and ending at the last node.
- The last node’s next pointer is set to NULL since it doesn’t point to any node.
Representation of a Singly Linked List
Below is the representation of a node in a singly linked list in multiple programming languages:
C:
struct node {
int data; // Data part
struct node *next; // Pointer to the next node
};
C++:
struct node {
int data; // Data part
node* next; // Pointer to the next node (note: node* is used instead of struct node*)
};
C#:
class Node {
public int data; // Data part
public Node next; // Pointer to the next node
}
Java:
class Node {
int data; // Data part
Node next; // Pointer to the next node
}
Python:
class Node:
def __init__(self, data):
self.data = data # Data part
self.next = None # Pointer to the next node
Key Differences
- C: Uses
struct
to define a node, with explicit declaration of a pointer to the next node (struct node* next
). - C++: Similar to C, but
struct
andclass
in C++ are nearly identical, sonode* next
is commonly used instead ofstruct node* next
. - C#: A class is used with explicit access modifiers (
public
). - Java: Follows object-oriented design using a class with
public
fields. - Python: Uses
class
and the constructor__init__
to initialize node attributes.
Each language utilizes its own syntax for pointers, memory management, and object handling, but the concept of a node in a linked list remains consistent.
Example
Consider three nodes with addresses 100, 200, and 300. Their representation in a singly linked list would look like this:
Address | Data | Next Pointer |
---|---|---|
100 | 1 | 200 |
200 | 2 | 300 |
300 | 3 | NULL |
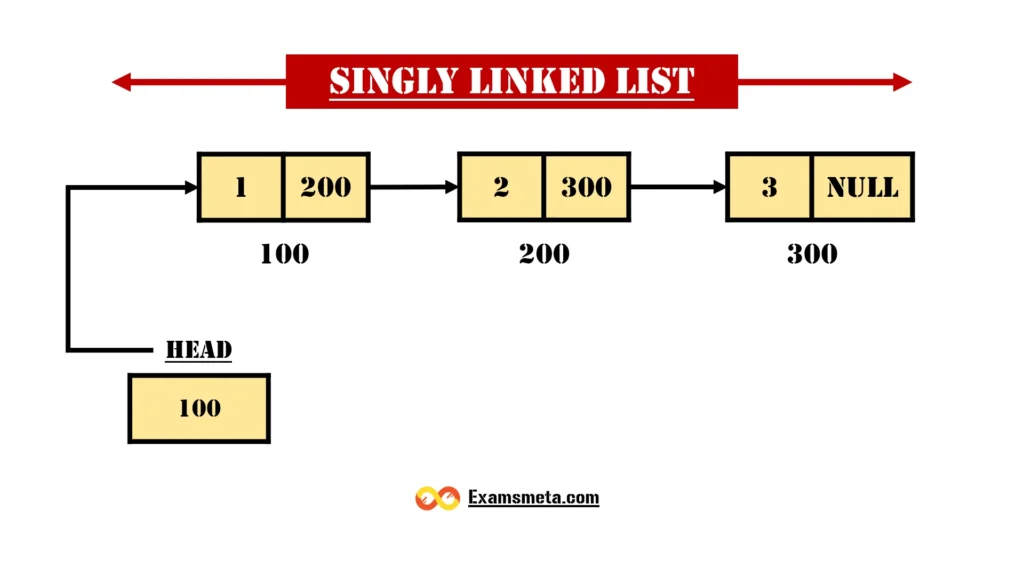
We can observe from the above Figure, there are three distinct nodes with addresses 100, 200, and 300, respectively. The first node contains the address of the next node (200), the second node holds the address of the final node (300), and the third node has its address part set to NULL, indicating that it does not point to any other node.
The pointer that stores the address of the initial node is referred to as the head pointer.
This structure represents a singly linked list, characterized by its single link between nodes. In this type of list, traversal is only possible in the forward direction, as there is no link to traverse backward.
Use Case
- Used in applications like stacks, queues, and basic dynamic memory manipulation.
2. Doubly Linked List
A doubly linked list extends the functionality of a singly linked list by adding a previous pointer to each node, allowing traversal in both directions.
Characteristics
- Two Pointers: Each node contains:
- Next Pointer: Points to the next node.
- Previous Pointer: Points to the previous node.
- Traversal can be done forwards or backward.
Representation of a Doubly Linked List
Here’s how you can define a doubly linked list node (which contains both a pointer to the next and previous nodes) in C, C++, C#, Java, and Python:
C:
struct node {
int data; // Data part
struct node *next; // Pointer to the next node
struct node *prev; // Pointer to the previous node
};
C++:
struct node {
int data; // Data part
node* next; // Pointer to the next node
node* prev; // Pointer to the previous node
};
C#:
class Node {
public int data; // Data part
public Node next; // Pointer to the next node
public Node prev; // Pointer to the previous node
}
Java:
class Node {
int data; // Data part
Node next; // Pointer to the next node
Node prev; // Pointer to the previous node
}
Python:
class Node:
def __init__(self, data):
self.data = data # Data part
self.next = None # Pointer to the next node
self.prev = None # Pointer to the previous node
Key Differences
- C: Uses
struct
to define a node, where pointers to both the next and previous nodes are explicitly declared (struct node* next
,struct node* prev
). - C++: Similar to C but uses
node* next
andnode* prev
to point to the next and previous nodes, respectively. - C#: The
class
keyword is used, andNode
is typically declared aspublic
for access to its members. - Java: Uses a
class
structure with fieldsnext
andprev
pointing to the next and previous nodes. - Python: Defines a class with an
__init__
constructor, wherenext
andprev
are initialized toNone
.
The implementation of a doubly linked list node is almost the same in all languages, with some minor differences in syntax and memory management. In all cases, you have the data, next pointer, and prev pointer for each node.
Example
Three nodes with addresses 100, 200, and 300 are represented as:
Address | Previous Pointer | Data | Next Pointer |
---|---|---|---|
100 | NULL | 1 | 200 |
200 | 100 | 2 | 300 |
300 | 200 | 3 | NULL |
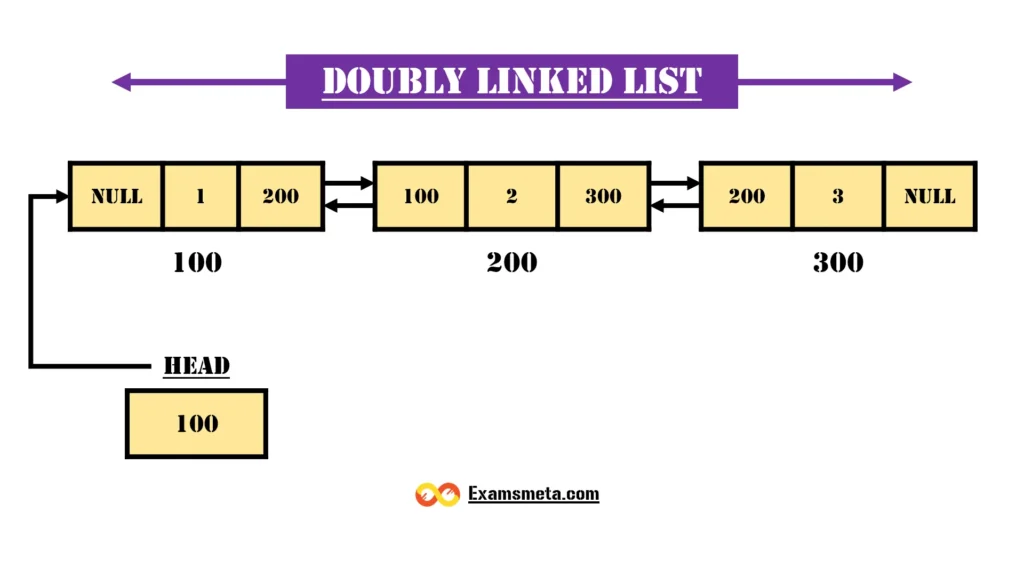
From the above figure we can observe that, In a doubly linked list, each node contains two address parts: one part stores the address of the next node, and the other stores the address of the previous node. This bidirectional connection allows traversal in both forward and backward directions. The first node in the list, known as the head node, has its previous address part set to NULL, indicating that there is no node before it. Similarly, the last node in the list has its next address part set to NULL, marking the end of the list.
Use Case
- Efficient in applications requiring bidirectional traversal, such as navigation systems and undo/redo operations.
3. Circular Linked List
A circular linked list is a variation of the singly linked list where the last node’s next pointer points back to the head node, forming a circular structure.
Characteristics
- No NULL Pointer: The last node links to the first node, creating a continuous loop.
- Traversal: Can theoretically continue indefinitely due to its circular nature.
Representation of a Circular Linked List
The C structure is similar to a singly linked list:
struct node {
int data;
struct node *next;
};
Example
Three nodes (100, 200, 300) form a circular linked list as:
Address | Data | Next Pointer |
---|---|---|
100 | 5 | 200 |
200 | 6 | 300 |
300 | 7 | 100 |
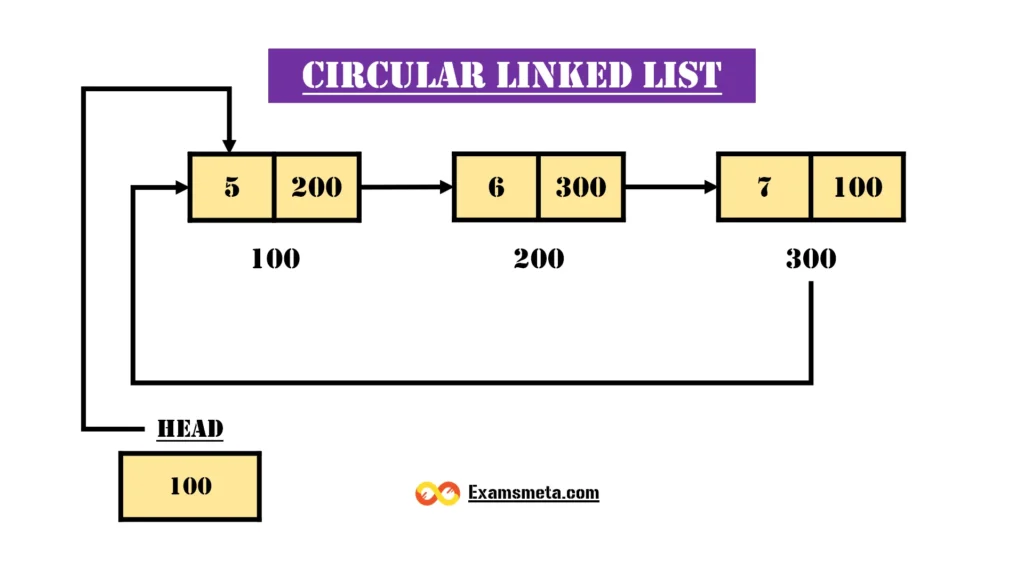
Use Case
- Commonly used in applications requiring continuous looping, such as multiplayer games and scheduler algorithms.
4. Doubly Circular Linked List
A doubly circular linked list combines the features of a doubly linked list and a circular linked list, forming a structure where:
- The last node’s next pointer points to the first node.
- The first node’s previous pointer points to the last node.
Characteristics
- No NULL Pointers: Both previous and next pointers form a continuous loop.
- Bidirectional Traversal: Supports both forward and backward navigation.
Representation of a Doubly Circular Linked List
The C structure is similar to a doubly linked list:
struct node {
int data;
struct node *next;
struct node *prev;
};
Example
Three nodes (100, 200, 300) are represented as:
Address | Previous Pointer | Data | Next Pointer |
---|---|---|---|
100 | 300 | 1 | 200 |
200 | 100 | 2 | 300 |
300 | 200 | 3 | 100 |
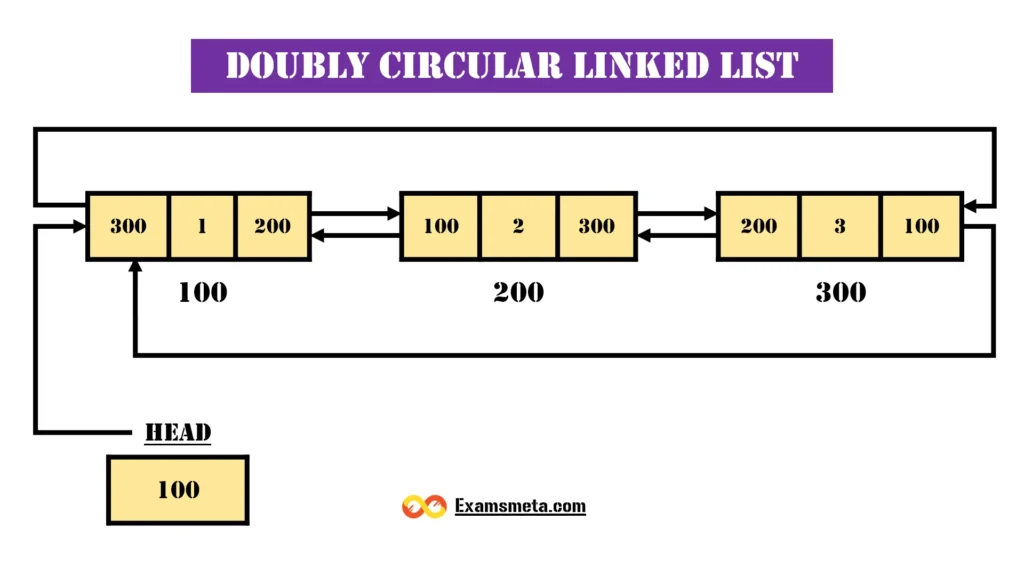
We can observe from the above figure, In a doubly linked list, each node has two address parts: one storing the address of the next node and the other storing the address of the previous node. The first node has its previous address set to NULL, indicating no preceding node. However, in a doubly circular linked list, the structure is modified so that the last node connects back to the first node, forming a continuous loop.
This type of list retains the bidirectional nature of the doubly linked list, with each node still pointing to both its predecessor and successor, but unlike a standard doubly linked list, it does not contain NULL in the previous field of the first node or the next field of the last node. With its three components—data, next address, and previous address—it mirrors the structure of a doubly linked list while providing a circular connection for uninterrupted traversal.
Use Case
- Used in real-time applications like music playlists and navigation menus.
Key Differences Between Linked List
Feature | Singly Linked List | Doubly Linked List | Circular Linked List | Doubly Circular Linked List |
---|---|---|---|---|
Traversal | Forward only | Forward and backward | Forward | Forward and backward |
End Node | Points to NULL | Points to NULL | Points to the first node | Points to the first node |
Memory Usage | Lower | Higher (extra pointer) | Similar to singly | Higher (extra pointer) |
Complexity | Lower | Higher | Similar to singly | Higher |
Advantages of Linked Lists
- Dynamic Memory Allocation: Allows memory to be allocated as needed.
- Efficient Insertion/Deletion: Operations can be performed in O(1) time at known positions.
- No Wastage: Unlike arrays, linked lists do not require pre-defined memory allocation.
Disadvantages of Linked Lists
- Extra Memory Overhead: Storing pointer information increases memory usage.
- Sequential Access: Accessing elements requires traversal, unlike arrays which offer random access.
- Complex Implementation: Pointers increase implementation complexity.
Practical Applications of Linked Lists
- Dynamic Memory Allocation: Used in malloc(), calloc(), and other heap-related operations.
- Stack and Queue Implementations: Linked lists provide efficient alternatives to array-based stacks and queues.
- Graph Representation: Adjacency lists in graph algorithms use linked lists.
- Operating Systems: Task scheduling and memory allocation use variations of circular and doubly linked lists.
- Text Editors: Undo functionality relies on doubly linked lists.
Conclusion
Linked lists are a versatile and essential data structure in computer science, providing a foundation for more complex structures like trees and graphs. Understanding the differences between singly linked lists, doubly linked lists, circular linked lists, and doubly circular linked lists allows developers to select the appropriate type for their applications. With proper implementation and optimization, linked lists can significantly enhance program performance and flexibility.
Frequently Asked Questions (FAQs)
What is a linked list, and how does it differ from an array?
A linked list is a linear data structure where each element, called a node, contains two parts:
- Data: Stores the actual value.
- Pointer: Holds the address of the next node.
In contrast, an array is a collection of elements stored in contiguous memory locations.
Key Differences:
- Memory Allocation: Linked lists use dynamic memory allocation, whereas arrays require static memory allocation.
- Size Flexibility: Linked lists can grow or shrink dynamically, while arrays have a fixed size.
- Traversal: Arrays allow random access using indices, but linked lists require sequential traversal.
What are the types of linked lists?
The four main types of linked lists are:
- Singly Linked List: Nodes contain data and a pointer to the next node. Traversal is unidirectional.
- Doubly Linked List: Each node has pointers to both the previous and the next nodes, allowing bidirectional traversal.
- Circular Linked List: Similar to a singly linked list, but the last node points back to the first node, forming a loop.
- Doubly Circular Linked List: Combines features of doubly and circular linked lists; the last node points to the first, and vice versa.
What are the advantages of linked lists over arrays?
Linked lists offer several advantages over arrays:
- Dynamic Size: The size of a linked list can change at runtime, unlike arrays with fixed size.
- Efficient Insertion/Deletion: Adding or removing elements (especially at the beginning or middle) is faster in linked lists as it only involves pointer adjustments.
- No Memory Wastage: Memory is allocated only when needed.
What is the role of the head pointer in a linked list?
The head pointer is a special pointer that holds the address of the first node in a linked list.
- It acts as the entry point for the list.
- Without the head pointer, the linked list becomes inaccessible.
- In a circular linked list, the head pointer helps identify the starting node even though the last node points back to the first node.
What is the difference between a singly and a doubly linked list?
Feature | Singly Linked List | Doubly Linked List |
---|---|---|
Number of Pointers | One (points to the next node). | Two (points to the next and previous nodes). |
Traversal | Only forward traversal is possible. | Allows both forward and backward traversal. |
Memory Usage | Uses less memory (one pointer per node). | Requires more memory (two pointers per node). |
Use Cases | Stacks, simple queues. | Navigation systems, undo/redo functionalities. |
What is a circular linked list, and where is it used?
A circular linked list is a linked list where the last node points back to the first node, forming a loop.
- Key Feature: There is no NULL pointer; the list has no defined start or end.
- Use Cases:
- Implementing round-robin scheduling in operating systems.
- Managing music playlists or other structures that require continuous looping.
What is the difference between a circular and a doubly circular linked list?
Feature | Circular Linked List | Doubly Circular Linked List |
---|---|---|
Pointees per Node | One pointer (next). | Two pointers (next and previous). |
Bidirectional Access | No (only forward traversal). | Yes (traversal in both directions). |
Applications | Simple looping tasks. | More complex tasks like bidirectional navigation. |
What are the practical applications of linked lists?
Linked lists are used in a variety of applications, including:
- Stacks and Queues: For dynamic data handling.
- Graphs: Representing adjacency lists.
- Dynamic Memory Allocation: Tracking free memory blocks (e.g., in malloc/free).
- Navigation Systems: In doubly linked lists, forward/backward traversal allows efficient data navigation.
- Music Players: Circular linked lists manage repeat playlists.
How do you implement a linked list in C?
Below is a basic implementation of a singly linked list in C:
#include <stdio.h>
#include <stdlib.h>
// Define the structure of a node
struct node {
int data;
struct node *next;
};
// Function to print the linked list
void printList(struct node *n) {
while (n != NULL) {
printf("%d -> ", n->data);
n = n->next;
}
printf("NULL\n");
}
int main() {
// Allocate memory for nodes
struct node *head = NULL;
struct node *second = NULL;
struct node *third = NULL;
head = (struct node *)malloc(sizeof(struct node));
second = (struct node *)malloc(sizeof(struct node));
third = (struct node *)malloc(sizeof(struct node));
// Assign data and link nodes
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = NULL;
// Print the list
printList(head);
return 0;
}
Output...
1 -> 2 -> 3 -> NULL
What are the disadvantages of linked lists?
While linked lists are versatile, they have some disadvantages:
- Memory Overhead: Each node requires extra memory for storing pointers.
- Sequential Access Only: Accessing elements requires traversing the list from the head.
- Complex Implementation: Managing pointers can introduce bugs, such as dangling pointers or memory leaks.
- Not Cache-Friendly: Unlike arrays, linked lists are not stored in contiguous memory locations, which can impact cache performance.
Why is dynamic memory allocation important in linked lists?
Dynamic memory allocation allows memory to be allocated at runtime, which is a significant advantage of linked lists over arrays.
- Reason: In a linked list, nodes are created and linked as needed, making it suitable for situations where the total number of elements is unknown at compile time.
- For example:
- In an array, unused memory remains wasted if the allocated size is too large.
- A linked list only allocates memory for nodes when they are added, minimizing memory wastage.
How is a node structured in a linked list?
A node is the fundamental building block of a linked list. It has two parts:
- Data Part: Stores the actual value or information.
- Pointer Part: Holds the memory address of the next (or sometimes previous) node.
Example in C:
For a singly linked list:
struct node {
int data; // Data part
struct node *next; // Pointer part
};
For a doubly linked list:
struct node {
int data;
struct node *next; // Pointer to the next node
struct node *prev; // Pointer to the previous node
};
How is a circular linked list advantageous in real-time systems?
A circular linked list is ideal for real-time systems because:
- Continuous Traversal: It loops back to the starting node, ensuring no node is ever inaccessible.
- Efficient Task Scheduling: In round-robin scheduling, each process (node) gets equal time in a circular manner.
- No End Condition: Avoids NULL checks, making traversal logic simpler in cyclic operations like buffer management or music playlists.
What is the time complexity of operations on a linked list?
The time complexity for common operations in a linked list depends on the type of operation:
- Insertion at the Head: O(1) (constant time).
- Insertion at the Tail:
- O(n) for singly linked lists (traversal required).
- O(1) for doubly linked lists if the tail pointer is maintained.
- Search for an Element: O(n) (linear traversal).
- Deletion:
- O(1) if the node is already located.
- O(n) if traversal is required to locate the node.
What is the significance of the NULL pointer in linked lists?
The NULL pointer indicates the end of a linked list or the absence of a successor node.
- In a singly linked list or doubly linked list, the last node’s pointer is set to NULL.
- In circular linked lists, there is no NULL pointer, as the last node points back to the head node.
How do you reverse a singly linked list?
Reversing a singly linked list involves changing the direction of the pointers between nodes.
Steps:
- Initialize three pointers: prev = NULL, current = head, and next = NULL.
- Iterate through the list:
- Store the next node:
next = current->next
. - Reverse the pointer:
current->next = prev
. - Move the pointers forward:
prev = current
,current = next
.
- Store the next node:
- Set the head to the last node:
head = prev
.
Code Example in C:
void reverseList(struct node** head_ref) {
struct node* prev = NULL;
struct node* current = *head_ref;
struct node* next = NULL;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
*head_ref = prev;
}
What are the limitations of circular linked lists?
Despite their advantages, circular linked lists have some limitations:
- Infinite Loop Risk: Improper traversal logic can lead to an infinite loop due to the circular nature of the list.
- Complex Deletion: Deleting the last node requires a traversal to locate it, increasing time complexity.
- Memory Overhead: Maintaining a circular structure might require additional logic and memory.
What are sentinel nodes in linked lists?
A sentinel node is a special dummy node used to simplify certain operations in a linked list.
- Purpose: Acts as a placeholder to eliminate edge cases like handling empty lists or the first node differently.
- Example:
- In circular linked lists, a sentinel node can serve as the head, making it easier to manage insertions and deletions consistently.
How are linked lists used in graph representation?
Graphs can be represented using linked lists in the form of an adjacency list.
- Adjacency List Representation:
- Each vertex is represented as a node in a list.
- Each node points to a linked list containing its adjacent vertices.
Example:
For a graph with vertices A, B, and C, where A → B, A → C, the adjacency list would be:
Vertex | Adjacency List |
---|---|
A | B → C → NULL |
B | NULL |
C | NULL |
What are memory leaks in linked lists, and how can they be avoided?
A memory leak occurs when dynamically allocated memory is not properly deallocated, resulting in unused memory that cannot be reclaimed.
Causes in Linked Lists:
- Failing to free a node after deleting it.
- Losing the reference to a node during pointer adjustments.
Prevention:
- Use the
free()
function in C to deallocate the memory of deleted nodes. - Traverse the list and free each node before exiting the program:
void freeList(struct node* head) {
struct node* temp;
while (head != NULL) {
temp = head;
head = head->next;
free(temp);
}
}