Arrays are fundamental data structures in programming and are extensively used due to their simplicity and efficiency in managing collections of elements. Understanding how arrays are stored in memory is crucial for optimizing programs, particularly in terms of speed and memory usage. This article provides an in-depth look at memory allocation for arrays, covering topics such as indexing, memory addresses, data alignment, and practical examples in various programming languages. We’ll explore array creation, indexing, accessing, and managing elements in detail.
Table of Contents
Introduction to Arrays and Memory Allocation
An array is a collection of elements stored in contiguous memory locations. This property enables direct access to any element in constant time using its index. When an array is declared, memory is allocated for each of its elements in a contiguous block, which allows the processor to efficiently access and manipulate the elements. The starting point, or the base address, is the memory address of the first element, and each subsequent element is positioned immediately after the previous one in memory.
Indexing in Arrays
Indexing allows accessing each element within the array. Different languages or systems might follow various indexing conventions, which are:
- 0-Based Indexing: This is the most common indexing method, where the first element has an index of 0. Therefore, an array of size 5 will have indices from 0 to 4.
- 1-Based Indexing: Some languages or applications use 1-based indexing, where the first element has an index of 1. The indices in a 5-element array would then range from 1 to 5.
- n-Based Indexing: In this type, the starting index can be any arbitrary value. This is generally seen in certain mathematical applications or specialized programming environments.
In this article, we’ll be focusing on 0-based indexing, as it is commonly used in languages like C, C++, Java, and Python.
Memory Allocation in Arrays: Key Concepts
- Contiguous Memory Allocation: Since arrays are stored in contiguous memory locations, the address of each element can be determined if the base address is known.
- Data Type Size: The size of each element in an array depends on the data type. For instance, an
int
in C or C++ often takes 4 bytes, while afloat
might also take 4 bytes, depending on the system. - Formula for Address Calculation: The address of an element at index
i
can be calculated as:
$$
\text{address of arr[i]} $$ $$ = \text{base address} + i \times \text{size of each element}
$$
For example, if the base address of an integer array arr
is 100
and each integer occupies 4 bytes
, the address of arr[3]
will be 100 + (3 * 4) = 112
.
Memory Allocation Example for an Integer Array
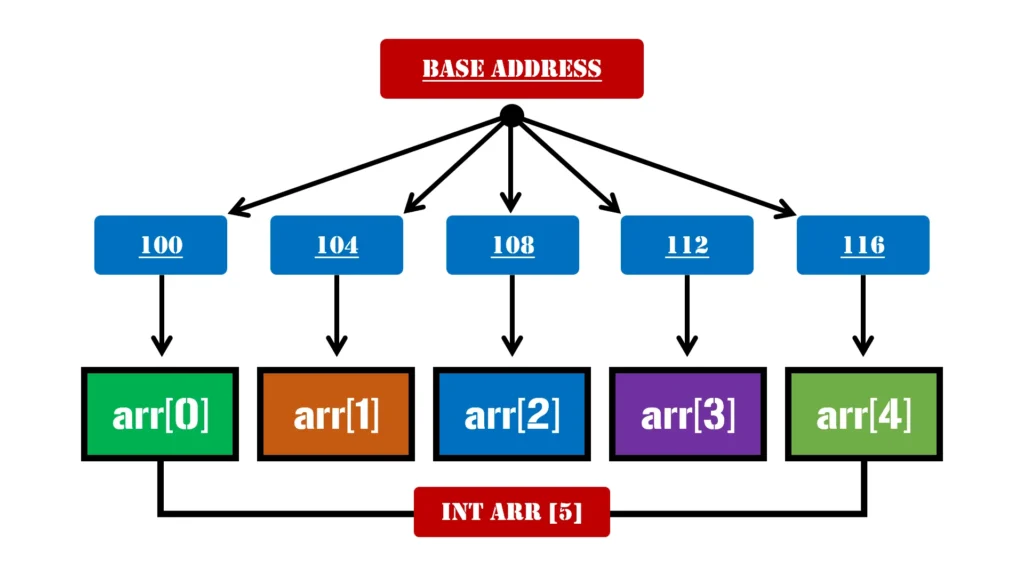
Let’s consider an integer array arr
of size 5 with a base address of 100, where each integer takes 4 bytes in memory.
Index | Element | Memory Address |
---|---|---|
0 | arr[0] | 100 |
1 | arr[1] | 104 |
2 | arr[2] | 108 |
3 | arr[3] | 112 |
4 | arr[4] | 116 |
With this understanding, let’s dive into code examples across various programming languages.
Examples of Array Memory Allocation in Various Programming Languages
Below are examples demonstrating the memory allocation of arrays in C, C++, C#, Java, and Python. Each example illustrates how elements are stored, accessed, and managed.
1. C Program Example
#include <stdio.h>
int main() {
int arr[5] = {10, 20, 30, 40, 50};
printf("Base address of arr: %p\n", (void*)arr);
for (int i = 0; i < 5; i++) {
printf("Address of arr[%d] = %p, Value = %d\n", i, (void*)&arr[i], arr[i]);
}
return 0;
}
Explanation:
int arr[5] = {10, 20, 30, 40, 50};
declares an integer arrayarr
with 5 elements.printf
statements output the base address and each element’s address and value.
Output:
Base address of arr: 0x7ffd23d3e890
Address of arr[0] = 0x7ffd23d3e890, Value = 10
Address of arr[1] = 0x7ffd23d3e894, Value = 20
Address of arr[2] = 0x7ffd23d3e898, Value = 30
Address of arr[3] = 0x7ffd23d3e89c, Value = 40
Address of arr[4] = 0x7ffd23d3e8a0, Value = 50
2. C++ Program Example
#include <iostream>
using namespace std;
int main() {
int arr[5] = {10, 20, 30, 40, 50};
cout << "Base address of arr: " << &arr[0] << endl;
for (int i = 0; i < 5; i++) {
cout << "Address of arr[" << i << "] = " << &arr[i] << ", Value = " << arr[i] << endl;
}
return 0;
}
Explanation:
- Similar to the C example, this code uses
cout
to display the base address, element addresses, and values in C++.
3. Java Program Example
public class ArrayMemoryAllocation {
public static void main(String[] args) {
int[] arr = {10, 20, 30, 40, 50};
System.out.println("Base address of arr (estimated): " + arr.hashCode());
for (int i = 0; i < arr.length; i++) {
System.out.println("Value at arr[" + i + "] = " + arr[i]);
}
}
}
Explanation:
- In Java, the exact memory address is not accessible, but we can use the
hashCode()
method as an approximation of the base address.
4. Python Program Example
import numpy as np
arr = np.array([10, 20, 30, 40, 50])
print("Base address of arr:", arr.__array_interface__['data'][0])
for i in range(len(arr)):
print(f"Value at arr[{i}] = {arr[i]}, Estimated memory location: {arr.__array_interface__['data'][0] + i * arr.itemsize}")
Explanation:
__array_interface__['data'][0]
provides an estimate of the array’s memory location in Python (using NumPy for demonstration).- This example also calculates each element’s memory offset based on the item size.
Conclusion
Understanding array memory allocation can aid in optimizing code, especially in performance-sensitive applications. By knowing how arrays occupy contiguous memory and the mechanics of base address and indexing, programmers can effectively design memory-efficient solutions.
Frequently Asked Questions (FAQs)
What is an array, and how is it stored in memory?
An array is a data structure that stores a collection of elements of the same data type in contiguous memory locations. This contiguous allocation means that each element in an array is stored next to the other, allowing for efficient access and manipulation. Arrays are accessed using indices, which act as pointers to specific memory locations.
For example, if we declare an integer array int arr[5]
in C, and the base address (or starting address) is 100 bytes, the array elements are stored sequentially in memory as follows:
Element | Memory Address |
---|---|
arr[0] | 100 |
arr[1] | 104 |
arr[2] | 108 |
arr[3] | 112 |
arr[4] | 116 |
Each element is spaced based on the size of the data type. Here, an int
typically occupies 4 bytes.
What are the different types of indexing used in arrays?
Arrays can use different indexing methods to access elements:
- 0-Based Indexing: The first element has an index of 0. For instance, in languages like C, C++, Java, and Python, if we declare
int arr[5]
, thenarr[0]
is the first element. - 1-Based Indexing: Here, the first element is at index 1, commonly seen in mathematics or specific programming languages and database systems. For example,
arr[1]
represents the first element. - n-Based Indexing: This is more flexible and allows the starting index to be any arbitrary integer, such as
5
or10
. It’s usually implemented in certain data applications or numerical analysis environments.
0-based indexing is widely used in most programming languages due to its efficiency in pointer arithmetic and memory addressing.
How does memory allocation work for arrays in languages like C and C++?
In C and C++, memory for arrays is allocated in a contiguous block. The base address of the array (the address of the first element) is used to calculate the address of any other element in the array.
For an integer array int arr[5]
with a base address of 100 bytes, the address of any element arr[i]
is calculated as:
$$
\text{address of arr[i]} $$ $$ = \text{base address} + i \times \text{size of int}
$$
This formula allows fast, constant-time access to any element by directly calculating its address, which is crucial for low-level programming and system optimization.
What are the advantages of using arrays in programming?
Arrays offer several key benefits:
- Direct Access: Since elements are stored in contiguous memory, any element can be accessed in constant time using its index. This makes operations like retrieval and updating extremely efficient.
- Efficient Memory Usage: Arrays have a fixed size, meaning memory is allocated only once. This is useful for managing memory in embedded systems or real-time applications.
- Ease of Use: They simplify handling multiple related elements, as elements are organized sequentially. Arrays are essential in implementing more complex data structures like stacks, queues, and hash tables.
- Indexing Flexibility: Arrays can be traversed using loops (such as
for
orwhile
), making them straightforward to manipulate in bulk.
However, arrays have a fixed size, so the number of elements must be known in advance. This can lead to wasted memory if the array size is overestimated or out-of-bounds errors if underestimated.
How does indexing work in Python arrays or lists, and how is memory managed differently than in C/C++?
In Python, the list data type is often used as a dynamic array. Unlike C or C++ arrays, Python lists are more flexible because they allow elements of different data types and can grow or shrink as needed.
Python lists are 0-indexed (the first element is at index 0). However, Python’s memory management is different:
- Dynamic Resizing: Lists in Python can change in size. When an element is added, Python often allocates more memory than necessary, preparing for future additions. If the list exceeds its current capacity, Python reallocates a larger block of memory.
- Memory References: Python lists don’t store elements directly in contiguous memory. Instead, they hold references (or pointers) to the actual objects in memory. This enables flexibility but may result in slower access times compared to statically typed arrays.
For example, if my_list = [1, 2, 3]
, the elements in my_list
are stored at different memory locations, but the list itself holds references to these memory addresses.
How can we calculate the address of an element in an array?
To calculate the memory address of an array element, we need the base address, the index of the element, and the size of each element. The formula to calculate the address of arr[i]
is:
$$
\text{address of arr[i]} $$ $$ = \text{base address} + i \times \text{size of each element}
$$
For instance, in a C program, if arr[5]
is an integer array with a base address of 100 bytes and an integer size of 4 bytes, the address of arr[3]
is calculated as:
$$
\text{address of arr[3]} = 100 + 3 \times 4 = 112
$$
This calculation ensures that each element in an array can be accessed directly, a key feature of arrays in system-level programming.
Why is 0-based indexing widely used in programming languages?
0-based indexing is popular due to its efficiency in pointer arithmetic and memory management. When using 0-based indexing:
- Base Address Efficiency: The address of the first element
arr[0]
is exactly the base address, simplifying calculations and saving a single addition operation. - Mathematical Consistency: Many mathematical operations and algorithms (such as iteration in loops) align naturally with 0-based indexing. For instance, in a loop,
for(int i = 0; i < n; i++)
, we can efficiently access elements fromarr[0]
toarr[n-1]
. - Memory Efficiency: In low-level programming (such as assembly), 0-based indexing often aligns better with hardware addressing, optimizing memory access speed.
Most popular languages, including C, C++, Java, and Python, use 0-based indexing for these reasons.
How does array size impact memory allocation and program efficiency?
The size of an array affects both memory usage and program performance. A larger array means more memory consumption, while a smaller array conserves memory but may require more frequent memory management if dynamically resized.
- Static Arrays: In languages like C and C++, arrays have a fixed size. This can be beneficial in terms of memory predictability but may lead to wasted memory if the array is underutilized.
- Dynamic Arrays: Languages like Python and Java use dynamic arrays that can grow or shrink. Dynamic arrays allocate additional memory as needed, which may involve reallocation (copying the array to a larger memory block), affecting performance.
Choosing an appropriate array size is crucial, especially in embedded systems or applications with limited resources.
What is an out-of-bounds error, and why does it occur in arrays?
An out-of-bounds error occurs when a program attempts to access an element outside the defined range of the array. In languages like C and C++, accessing out-of-bounds elements can cause undefined behavior, potentially leading to:
- Memory corruption: Overwriting unrelated data in memory.
- Program crashes: Attempting to access restricted memory.
- Security vulnerabilities: Malicious actors may exploit these errors to execute arbitrary code.
In Python and Java, out-of-bounds access raises an IndexError or ArrayIndexOutOfBoundsException, preventing unsafe memory access.
How can I print the memory addresses of array elements in different programming languages?
Printing memory addresses helps understand how arrays are allocated. Here’s a sample in C and Python:
C Example
#include <stdio.h>
int main() {
int arr[3] = {10, 20, 30};
for (int i = 0; i < 3; i++) {
printf("Address of arr[%d] = %p, Value = %d\n", i, (void*)&arr[i], arr[i]);
}
return 0;
}
Python Example (Using NumPy)
import numpy as np
arr = np.array([10, 20, 30])
for i in range(len(arr)):
print(f"Address of arr[{i}] = {arr.__array_interface__
['data'][0] + i * arr.itemsize}, Value = {arr[i]}")
These examples help visualize array addresses and illustrate how each element is spaced in memory based on its data type size.