In the world of computer science, data structures play a crucial role in organizing and managing information effectively. Among the various types of data structures, a doubly linked list stands out for its bidirectional navigation capability, where each node contains pointers to both its previous and next nodes. This article delves into the detailed process of inserting a new node at the end of a doubly linked list, a fundamental operation often encountered in programming.
Table of Contents
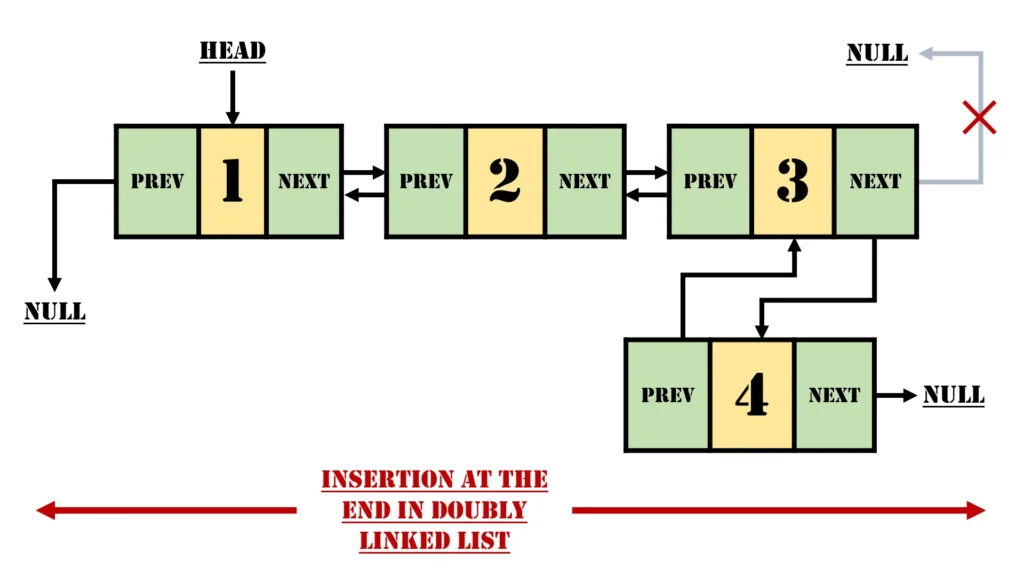
Understanding the Basics of a Doubly Linked List
A doubly linked list is a type of linked list where each node contains three components:
- A data field, which stores the actual information.
- A pointer to the previous node, often called
prev
. - A pointer to the next node, often called
next
.
Unlike a singly linked list, where traversal is limited to one direction (from the head to the tail), a doubly linked list allows navigation both forward and backward. This additional flexibility, however, comes at the cost of increased memory usage and slightly more complex algorithms.
Steps to Insert a Node at the End of a Doubly Linked List
Inserting a new node at the end of a doubly linked list involves specific steps. These steps ensure the list maintains its structure and allows seamless navigation after the insertion.
Step 1: Handle the Empty List Case
When the doubly linked list is empty (i.e., the head of the list is NULL
or None
), the new node becomes the head of the list.
- Create a new node:
- Allocate memory for the new node.
- Set its
data
field to the desired value. - Set both its
prev
andnext
pointers toNULL
orNone
, as it is the only node in the list.
- Update the head:
- Assign the new node as the head of the list, making it the starting point for all future traversals.
This scenario is relatively straightforward, as there are no other nodes to consider.
Step 2: Traverse the List to Find the Last Node
If the list is not empty, we need to traverse the list to locate the current last node. This involves the following substeps:
- Start at the head:
- Initialize a pointer, often called
curr
, to the head of the list.
- Initialize a pointer, often called
- Iterate through the list:
- Use a loop to move the
curr
pointer from one node to the next by following thenext
pointers. - Continue until you reach a node where
curr->next
(orcurr.next
) isNULL
orNone
. - This node is the current last node of the list.
- Use a loop to move the
Traversal is a fundamental aspect of working with linked lists, as it enables access to specific nodes without a direct indexing mechanism like arrays.
Step 3: Insert the New Node
Once the last node is identified, the insertion process begins. This step involves linking the new node to the existing list and ensuring bidirectional connectivity.
- Create the new node:
- Allocate memory for the new node.
- Set its
data
field to the desired value. - Initialize its
prev
andnext
pointers as follows:prev
should point to the current last node (i.e.,curr
).next
should beNULL
orNone
, as the new node will become the last node.
- Update the current last node:
- Set the
next
pointer of the current last node (i.e.,curr
) to the new node, establishing the forward link.
- Set the
- Update the new node’s
prev
pointer:- Confirm that the
prev
pointer of the new node points back to the current last node, completing the bidirectional connection.
- Confirm that the
Advantages of Proper Node Insertion
The insertion process, when done correctly, ensures several benefits:
- Data Integrity: Maintaining proper links prevents data loss or corruption within the list.
- Ease of Traversal: By keeping the
prev
andnext
pointers correctly assigned, the list remains navigable in both directions. - Scalability: The list can grow dynamically, accommodating new nodes without the need for pre-defined sizes.
Code Implementation
Below is a Python implementation of the above steps for inserting a new node at the end of a doubly linked list:
class Node:
def __init__(self, data):
self.data = data
self.prev = None
self.next = None
class DoublyLinkedList:
def __init__(self):
self.head = None
def insert_at_end(self, data):
new_node = Node(data)
# Step 1: Handle the empty list
if not self.head:
self.head = new_node
return
# Step 2: Traverse to the last node
curr = self.head
while curr.next:
curr = curr.next
# Step 3: Insert the new node
curr.next = new_node
new_node.prev = curr
Implementation In Multiple Programming Languages
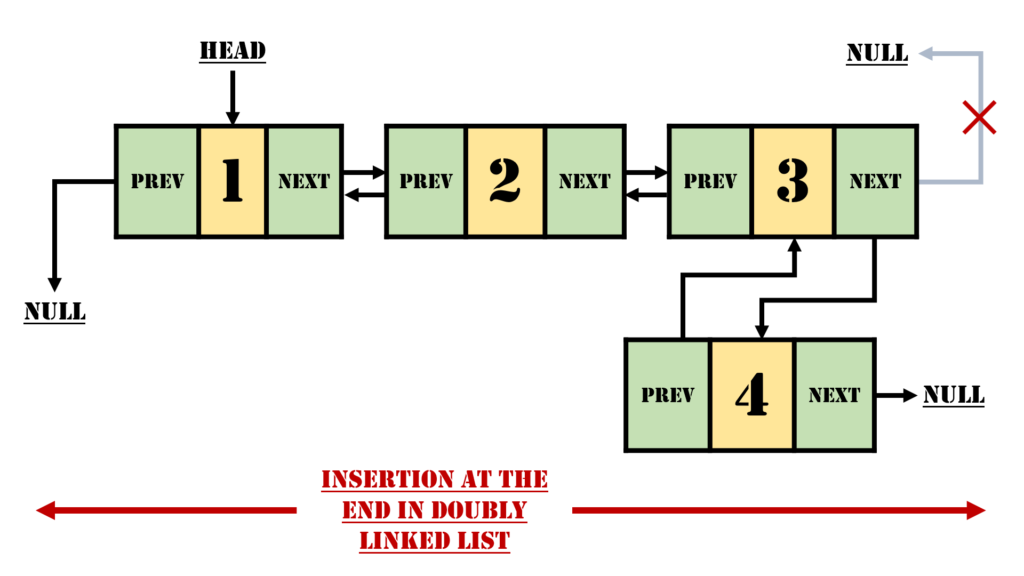
Here is the code written in C, C++, C#, Java, JavaScript, and Python, along with a detailed step-by-step explanation for each language.
C Program Implementation
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node *next;
struct Node *prev;
};
// Function to create a new node with the given data
struct Node *createNode(int new_data) {
struct Node *new_node = (struct Node *)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = NULL;
new_node->prev = NULL;
return new_node;
}
// Function to insert a new node at the end of the doubly linked list
struct Node* insertEnd(struct Node *head, int new_data) {
struct Node *new_node = createNode(new_data);
if (head == NULL) {
head = new_node;
} else {
struct Node *curr = head;
while (curr->next != NULL) {
curr = curr->next;
}
curr->next = new_node;
new_node->prev = curr;
}
return head;
}
// Function to print the linked list
void printList(struct Node *head) {
struct Node *curr = head;
while (curr != NULL) {
printf("%d ", curr->data);
curr = curr->next;
}
printf("\n");
}
int main() {
struct Node *head = createNode(1);
head->next = createNode(2);
head->next->prev = head;
head->next->next = createNode(3);
head->next->next->prev = head->next;
printf("Original Linked List: ");
printList(head);
printf("Inserting Node with data 4 at the end: ");
head = insertEnd(head, 4);
printList(head);
return 0;
}
C++ Implementation
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node* next;
Node* prev;
Node(int new_data) {
data = new_data;
next = nullptr;
prev = nullptr;
}
};
Node* insertEnd(Node* head, int new_data) {
Node* new_node = new Node(new_data);
if (head == nullptr) {
head = new_node;
} else {
Node* curr = head;
while (curr->next != nullptr) {
curr = curr->next;
}
curr->next = new_node;
new_node->prev = curr;
}
return head;
}
void printList(Node* head) {
Node* curr = head;
while (curr != nullptr) {
cout << curr->data << " ";
curr = curr->next;
}
cout << endl;
}
int main() {
Node* head = new Node(1);
head->next = new Node(2);
head->next->prev = head;
head->next->next = new Node(3);
head->next->next->prev = head->next;
cout << "Original Linked List: ";
printList(head);
cout << "Inserting Node with data 4 at the end: ";
head = insertEnd(head, 4);
printList(head);
return 0;
}
C# Implementation
using System;
class Node {
public int Data;
public Node Next;
public Node Prev;
public Node(int new_data) {
Data = new_data;
Next = null;
Prev = null;
}
}
class Program {
static Node InsertEnd(Node head, int new_data) {
Node newNode = new Node(new_data);
if (head == null) {
head = newNode;
} else {
Node curr = head;
while (curr.Next != null) {
curr = curr.Next;
}
curr.Next = newNode;
newNode.Prev = curr;
}
return head;
}
static void PrintList(Node head) {
Node curr = head;
while (curr != null) {
Console.Write(curr.Data + " ");
curr = curr.Next;
}
Console.WriteLine();
}
static void Main() {
Node head = new Node(1);
head.Next = new Node(2);
head.Next.Prev = head;
head.Next.Next = new Node(3);
head.Next.Next.Prev = head.Next;
Console.Write("Original Linked List: ");
PrintList(head);
Console.Write("Inserting Node with data 4 at the end: ");
head = InsertEnd(head, 4);
PrintList(head);
}
}
Java Implementation
class Node {
int data;
Node next, prev;
public Node(int new_data) {
data = new_data;
next = null;
prev = null;
}
}
public class DoublyLinkedList {
static Node insertEnd(Node head, int new_data) {
Node newNode = new Node(new_data);
if (head == null) {
head = newNode;
} else {
Node curr = head;
while (curr.next != null) {
curr = curr.next;
}
curr.next = newNode;
newNode.prev = curr;
}
return head;
}
static void printList(Node head) {
Node curr = head;
while (curr != null) {
System.out.print(curr.data + " ");
curr = curr.next;
}
System.out.println();
}
public static void main(String[] args) {
Node head = new Node(1);
head.next = new Node(2);
head.next.prev = head;
head.next.next = new Node(3);
head.next.next.prev = head.next;
System.out.print("Original Linked List: ");
printList(head);
System.out.print("Inserting Node with data 4 at the end: ");
head = insertEnd(head, 4);
printList(head);
}
}
JavaScript Implementation
class Node {
constructor(data) {
this.data = data;
this.next = null;
this.prev = null;
}
}
function insertEnd(head, new_data) {
let newNode = new Node(new_data);
if (head === null) {
head = newNode;
} else {
let curr = head;
while (curr.next !== null) {
curr = curr.next;
}
curr.next = newNode;
newNode.prev = curr;
}
return head;
}
function printList(head) {
let curr = head;
while (curr !== null) {
process.stdout.write(curr.data + " ");
curr = curr.next;
}
console.log();
}
let head = new Node(1);
head.next = new Node(2);
head.next.prev = head;
head.next.next = new Node(3);
head.next.next.prev = head.next;
console.log("Original Linked List: ");
printList(head);
let data = 4;
head = insertEnd(head, data);
console.log("Inserting Node with data 4 at the end: ");
printList(head);
Python Implementation
class Node:
def __init__(self, data):
self.data = data
self.next = None
self.prev = None
def insert_end(head, new_data):
new_node = Node(new_data)
if head is None:
head = new_node
else:
curr = head
while curr.next is not None:
curr = curr.next
curr.next = new_node
new_node.prev = curr
return head
def print_list(head):
curr = head
while curr is not None:
print(curr.data, end=" ")
curr = curr.next
print()
head = Node(1)
head.next = Node(2)
head.next.prev = head
head.next.next = Node(3)
head.next.next.prev = head.next
print("Original Linked List: ")
print_list(head)
data = 4
head = insert_end(head, data)
print("Inserting Node with data 4 at the end: ")
print_list(head)
Output
Original Linked List:
1 2 3
Inserting Node with data 4 at the end:
1 2 3 4
Time Complexity: O(1)
Auxiliary Space: O(1)
Summary
In all languages:
- A
Node
class/struct is defined to store the data, next pointer, and previous pointer. - A function
insertEnd()
is created to insert a new node at the end of the list. - A function
printList()
is used to print the list. - The new node with the value 4 is inserted at the end of the list.
- The updated list is printed in each case.
Conclusion
Understanding and implementing the process of inserting a new node at the end of a doubly linked list is an essential skill for programmers and data structure enthusiasts. This operation not only demonstrates the flexibility of linked lists but also reinforces the importance of careful pointer manipulation in dynamic data structures. By mastering such techniques, you pave the way for solving more complex problems in algorithms and software development.
So, next time you’re working with linked lists, remember the key steps: handle the empty list, traverse to the last node, and ensure bidirectional connectivity during insertion. Happy coding!
Related Articles
- Insertion in Doubly Linked List with Implementation: A Detailed Exploration
- Inserting a Node at the Beginning of a Doubly Linked List: A Detailed Exploration
- Inserting a Node After a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Inserting a New Node at the End of a Doubly Linked List: A Detailed Exploration
Read More Articles
- Data Structure (DS) Array:
- Why the Analysis of Algorithms is Important?
- Worst, Average, and Best Case Analysis of Algorithms: A Comprehensive Guide
- Understanding Pointers in C Programming: A Comprehensive Guide
- Understanding Arrays in Data Structures: A Comprehensive Exploration
- Memory Allocation of an Array: An In-Depth Comprehensive Exploration
- Understanding Basic Operations in Arrays: A Comprehensive Guide
- Understanding 2D Arrays in Programming: A Comprehensive Guide
- Mapping a 2D Array to a 1D Array: A Comprehensive Exploration
- Data Structure Linked List:
- Understanding Linked Lists in Data Structures: A Comprehensive Exploration
- Types of Linked List: Detailed Exploration, Representations, and Implementations
- Understanding Singly Linked Lists: A Detailed Exploration
- Understanding Doubly Linked List: A Comprehensive Guide
- Operations of Doubly Linked List with Implementation: A Detailed Exploration
- Insertion in Doubly Linked List with Implementation: A Detailed Exploration
- Inserting a Node at the Beginning of a Doubly Linked List: A Detailed Exploration
- Inserting a Node After a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Inserting a New Node at the End of a Doubly Linked List: A Detailed Exploration
- Deletion in a Doubly Linked List with Implementation: A Comprehensive Guide
- Deletion at the Beginning in a Doubly Linked List: A Detailed Exploration
- Deletion after a given node in Doubly Linked List: A Comprehensive Guide
- Deleting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Deletion at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Deletion at the End in Doubly Linked List: A Comprehensive Exploration
Frequently Asked Questions (FAQs) on Inserting a Node at the End of a Doubly Linked List
What is a doubly linked list, and how does it differ from a singly linked list?
A doubly linked list is a type of linked list where each node contains three components:
- A data field that holds the value of the node.
- A prev pointer that links to the previous node.
- A next pointer that links to the next node.
The key difference between a doubly linked list and a singly linked list lies in the number of pointers each node has:
- In a singly linked list, each node contains only one pointer, which points to the next node. Traversal is unidirectional (from head to tail).
- In a doubly linked list, each node has two pointers (
prev
andnext
), allowing traversal in both directions (from head to tail and vice versa).
This bidirectional feature of doubly linked lists makes them more versatile but slightly more complex to implement and manage.
Why is inserting a node at the end of a doubly linked list a common operation?
Inserting a node at the end is a frequent operation because:
- It extends the list dynamically, allowing data to grow without predefined size limitations, unlike arrays.
- The end of the list often serves as a natural position for appending data, especially in scenarios like maintaining logs or queues.
- It demonstrates the ability of the doubly linked list to handle dynamic insertion operations efficiently.
By leveraging the next and prev pointers, inserting at the end ensures proper connectivity without disrupting the existing structure.
What happens if the doubly linked list is empty during insertion?
When the doubly linked list is empty (i.e., its head pointer is NULL
or None
):
- The new node is directly assigned as the head of the list.
- Both its
prev
andnext
pointers are set toNULL
(orNone
), making it the sole element in the list.
This scenario is straightforward because there are no existing nodes to traverse or link. It essentially initializes the list with the new node as its first element.
How do we traverse a doubly linked list to find the last node?
Traversing a doubly linked list involves starting at the head and moving through each node until the last node is reached. The steps are:
- Initialize a pointer (e.g.,
curr
) to the head of the list. - Use a loop to move
curr
to the next node by following thenext
pointer. - Continue until the current node’s
next
pointer isNULL
orNone
.
This traversal is necessary because linked lists do not have direct indexing like arrays. The last node is identified as the one whose next
pointer does not reference another node.
What are the key steps for inserting a new node at the end of a doubly linked list?
The key steps for inserting a node at the end are:
- Handle the empty list case:
- Assign the new node as the head if the list is empty.
- Traverse to the last node:
- Use the
next
pointers to navigate from the head to the last node.
- Use the
- Insert the new node:
- Set the
next
pointer of the current last node to the new node. - Update the
prev
pointer of the new node to point back to the current last node.
- Set the
These steps ensure that the list remains properly connected in both directions.
Why is pointer manipulation critical in a doubly linked list?
Pointer manipulation is the backbone of all operations in a doubly linked list because:
- Data Integrity: Incorrect pointers can lead to data loss or circular references, making the list inaccessible.
- Traversal: Both the
next
andprev
pointers must be correctly updated to ensure smooth forward and backward traversal. - Dynamic Changes: Inserting or deleting nodes requires careful adjustment of pointers to maintain the list’s structure.
By properly managing these pointers, we ensure that the doubly linked list remains functional and reliable.
How is the memory footprint of a doubly linked list different from a singly linked list?
A doubly linked list uses more memory than a singly linked list because:
- Each node in a doubly linked list contains an additional pointer (
prev
) compared to a singly linked list. - This extra pointer increases the memory required for each node.
However, the trade-off is the enhanced functionality of bidirectional traversal and easier deletion or insertion operations at both ends of the list.
Can you provide a Python example of inserting a node at the end of a doubly linked list?
Certainly! Below is a Python implementation:
class Node:
def __init__(self, data):
self.data = data
self.prev = None
self.next = None
class DoublyLinkedList:
def __init__(self):
self.head = None
def insert_at_end(self, data):
new_node = Node(data)
if not self.head: # Handle the empty list
self.head = new_node
return
curr = self.head
while curr.next: # Traverse to the last node
curr = curr.next
curr.next = new_node # Link the new node
new_node.prev = curr # Set the prev pointer
This code illustrates the core concepts of handling the empty list, traversal, and bidirectional linkage.
What are the advantages of using a doubly linked list over other data structures?
The advantages of a doubly linked list include:
- Bidirectional Traversal: Ability to navigate both forward and backward, unlike singly linked lists.
- Ease of Insertion and Deletion: Operations at both ends are more efficient due to the availability of
prev
pointers. - Dynamic Memory Allocation: No need to specify the size beforehand, unlike arrays.
These features make doubly linked lists particularly useful in scenarios like implementing deques, navigation systems and undo functionality in software.
Are there any limitations to using doubly linked lists?
While doubly linked lists are powerful, they do have limitations:
- Increased Memory Usage: The additional
prev
pointer in each node requires extra memory compared to singly linked lists. - Complexity of Implementation: Managing two pointers (next and prev) increases the complexity of operations.
- Traversal Overhead: Traversing the list, especially for large datasets, can be time-consuming compared to arrays with direct indexing.
Despite these limitations, the versatility of doubly linked lists makes them indispensable in specific applications.