Managing linked lists efficiently is a critical skill in computer science. A doubly linked list, with its nodes containing both prev
and next
pointers, offers versatile manipulation capabilities. One of the common operations performed on this data structure is deleting a node at the beginning. This operation can be executed with constant time complexity, O(1), as it does not require traversal of the entire list. Let us delve into the process in detail, breaking it into logical steps, code implementation, and practical examples.
Table of Contents
Understanding Doubly Linked Lists
A doubly linked list is a data structure where each node contains three components:
- Data: Stores the value of the node.
- Next Pointer: Points to the next node in the sequence.
- Previous Pointer: Points to the previous node in the sequence.
This dual-pointer structure makes doubly linked lists advantageous for bi-directional traversal and efficient insertions or deletions at either end. However, every operation must carefully maintain the pointers to ensure the structure’s integrity.
When deleting a node at the beginning of the list, it is crucial to update the head pointer and appropriately set the prev
pointer of the new head node to NULL
. The operation can be summarized in well-defined steps.
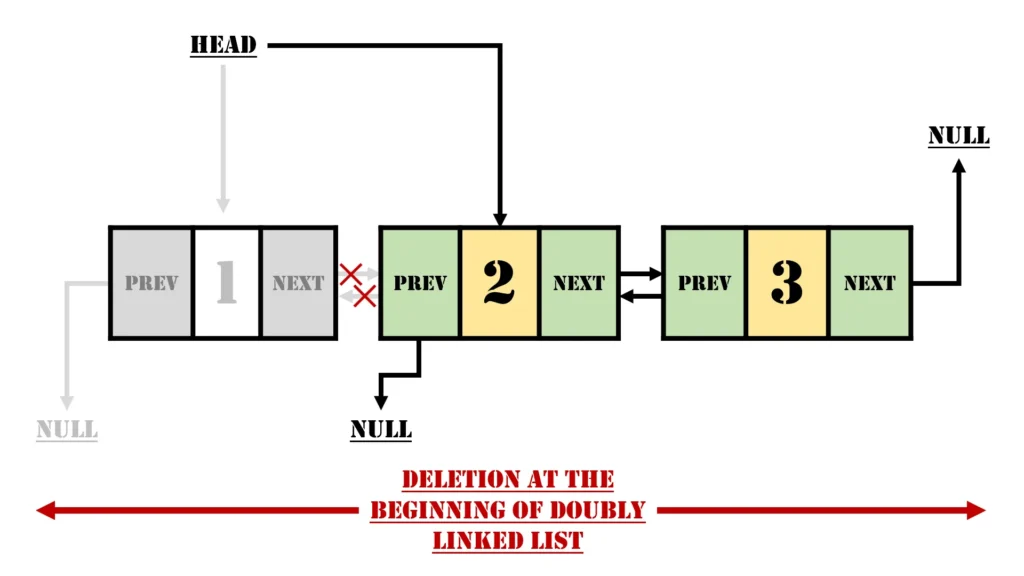
Steps to Delete the Head Node
The process of deleting the first node (head) of a doubly linked list involves the following steps:
- Check if the List is Empty
If the list is empty (head == NULL
), there is no node to delete. This condition should be handled gracefully to avoid runtime errors. - Store the Current Head Node in a Temporary Variable
A temporary pointer,temp
, is used to store the currenthead
node. This allows us to free its memory later without losing track of the list’s structure. - Update the Head Pointer
Move thehead
pointer to the next node (head = head->next
). This effectively removes the current head node from the list’s logical structure. - Set the Previous Pointer of the New Head to NULL
If the newhead
is notNULL
, itsprev
pointer must be set toNULL
(head->prev = NULL
). This step ensures the integrity of the new list. - Free Memory Allocated to the Old Head Node
Using thefree()
function in C, deallocate the memory for the old head node stored intemp
. - Return the New Head Pointer
Finally, return the updatedhead
pointer to the caller function.
Code Implementation
The following C program demonstrates how to delete the first node of a doubly linked list while adhering to the above steps.
#include <stdio.h>
#include <stdlib.h>
// Define the structure of a doubly linked list node
struct Node {
int data;
struct Node *prev;
struct Node *next;
};
// Function to delete the first node (head) of the list
struct Node *delHead(struct Node *head) {
// If the list is empty, return NULL
if (head == NULL)
return NULL;
// Store the current head in a temporary variable
struct Node *temp = head;
// Update the head pointer to the next node
head = head->next;
// If the new head is not NULL, set its prev pointer to NULL
if (head != NULL)
head->prev = NULL;
// Free memory allocated for the old head
free(temp);
// Return the new head pointer
return head;
}
// Function to print the doubly linked list
void printList(struct Node *head) {
struct Node *curr = head;
while (curr != NULL) {
printf("%d ", curr->data);
curr = curr->next;
}
printf("\n");
}
// Helper function to create a new node
struct Node *createNode(int data) {
struct Node *newNode = (struct Node *)malloc(sizeof(struct Node));
newNode->data = data;
newNode->prev = NULL;
newNode->next = NULL;
return newNode;
}
// Main function to demonstrate deletion
int main() {
// Create a hardcoded doubly linked list: 1 <-> 2 <-> 3
struct Node *head = createNode(1);
head->next = createNode(2);
head->next->prev = head;
head->next->next = createNode(3);
head->next->next->prev = head->next;
// Print the original list
printf("Original Linked List: ");
printList(head);
// Delete the head node
head = delHead(head);
// Print the updated list
printf("Updated Linked List: ");
printList(head);
return 0;
}
Coding Implementation In Multiple Programming Languages
Here is the code written in C, C++, C#, Java, JavaScript, and Python, along with a detailed step-by-step explanation for each language.
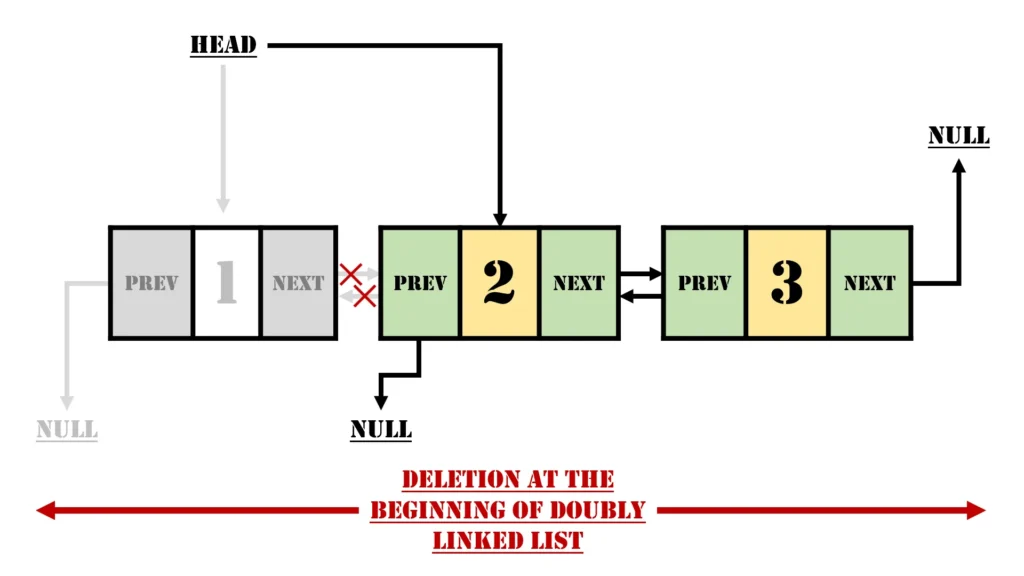
C Program Implementation
#include <stdio.h>
#include <stdlib.h>
// Define the structure of a doubly linked list node
struct Node {
int data;
struct Node *prev;
struct Node *next;
};
// Function to delete the first node (head) of the list
struct Node *delHead(struct Node *head) {
// If the list is empty, return NULL
if (head == NULL)
return NULL;
// Store the current head in a temporary variable
struct Node *temp = head;
// Update the head pointer to the next node
head = head->next;
// If the new head is not NULL, set its prev pointer to NULL
if (head != NULL)
head->prev = NULL;
// Free memory allocated for the old head
free(temp);
// Return the new head pointer
return head;
}
// Function to print the doubly linked list
void printList(struct Node *head) {
struct Node *curr = head;
while (curr != NULL) {
printf("%d ", curr->data);
curr = curr->next;
}
printf("\n");
}
// Helper function to create a new node
struct Node *createNode(int data) {
struct Node *newNode = (struct Node *)malloc(sizeof(struct Node));
newNode->data = data;
newNode->prev = NULL;
newNode->next = NULL;
return newNode;
}
// Main function to demonstrate deletion
int main() {
// Create a hardcoded doubly linked list: 1 <-> 2 <-> 3
struct Node *head = createNode(1);
head->next = createNode(2);
head->next->prev = head;
head->next->next = createNode(3);
head->next->next->prev = head->next;
// Print the original list
printf("Original Linked List: ");
printList(head);
// Delete the head node
head = delHead(head);
// Print the updated list
printf("Updated Linked List: ");
printList(head);
return 0;
}
C++ Implementation
#include <iostream>
using namespace std;
struct Node {
int data;
Node* prev;
Node* next;
};
// Function to delete the first node (head)
Node* delHead(Node* head) {
if (head == NULL)
return NULL;
Node* temp = head;
head = head->next;
if (head != NULL)
head->prev = NULL;
delete temp;
return head;
}
// Function to print the list
void printList(Node* head) {
Node* curr = head;
while (curr != NULL) {
cout << curr->data << " ";
curr = curr->next;
}
cout << endl;
}
// Helper function to create a new node
Node* createNode(int data) {
Node* newNode = new Node();
newNode->data = data;
newNode->prev = NULL;
newNode->next = NULL;
return newNode;
}
int main() {
Node* head = createNode(1);
head->next = createNode(2);
head->next->prev = head;
head->next->next = createNode(3);
head->next->next->prev = head->next;
cout << "Original Linked List: ";
printList(head);
head = delHead(head);
cout << "Updated Linked List: ";
printList(head);
return 0;
}
C# Implementation
using System;
class Node {
public int data;
public Node prev;
public Node next;
}
class Program {
// Function to delete the head node
static Node DelHead(Node head) {
if (head == null) return null;
Node temp = head;
head = head.next;
if (head != null)
head.prev = null;
return head;
}
// Function to print the list
static void PrintList(Node head) {
Node curr = head;
while (curr != null) {
Console.Write(curr.data + " ");
curr = curr.next;
}
Console.WriteLine();
}
// Helper function to create a new node
static Node CreateNode(int data) {
return new Node { data = data, prev = null, next = null };
}
static void Main(string[] args) {
Node head = CreateNode(1);
head.next = CreateNode(2);
head.next.prev = head;
head.next.next = CreateNode(3);
head.next.next.prev = head.next;
Console.WriteLine("Original Linked List: ");
PrintList(head);
head = DelHead(head);
Console.WriteLine("Updated Linked List: ");
PrintList(head);
}
}
Java Implementation
class Node {
int data;
Node prev, next;
Node(int data) {
this.data = data;
this.prev = null;
this.next = null;
}
}
public class DoublyLinkedList {
// Function to delete the head node
static Node delHead(Node head) {
if (head == null) return null;
Node temp = head;
head = head.next;
if (head != null)
head.prev = null;
return head;
}
// Function to print the list
static void printList(Node head) {
Node curr = head;
while (curr != null) {
System.out.print(curr.data + " ");
curr = curr.next;
}
System.out.println();
}
public static void main(String[] args) {
Node head = new Node(1);
head.next = new Node(2);
head.next.prev = head;
head.next.next = new Node(3);
head.next.next.prev = head.next;
System.out.println("Original Linked List: ");
printList(head);
head = delHead(head);
System.out.println("Updated Linked List: ");
printList(head);
}
}
JavaScript Implementation
class Node {
constructor(data) {
this.data = data;
this.prev = null;
this.next = null;
}
}
// Function to delete the head node
function delHead(head) {
if (!head) return null;
let temp = head;
head = head.next;
if (head) head.prev = null;
return head;
}
// Function to print the list
function printList(head) {
let curr = head;
while (curr) {
process.stdout.write(curr.data + " ");
curr = curr.next;
}
console.log();
}
// Helper function to create a new node
function createNode(data) {
return new Node(data);
}
// Main function to demonstrate deletion
let head = createNode(1);
head.next = createNode(2);
head.next.prev = head;
head.next.next = createNode(3);
head.next.next.prev = head.next;
console.log("Original Linked List: ");
printList(head);
head = delHead(head);
console.log("Updated Linked List: ");
printList(head);
Python Implementation
class Node:
def __init__(self, data):
self.data = data
self.prev = None
self.next = None
# Function to delete the head node
def del_head(head):
if head is None:
return None
temp = head
head = head.next
if head:
head.prev = None
return head
# Function to print the list
def print_list(head):
curr = head
while curr:
print(curr.data, end=" ")
curr = curr.next
print()
# Main function to demonstrate deletion
head = Node(1)
head.next = Node(2)
head.next.prev = head
head.next.next = Node(3)
head.next.next.prev = head.next
print("Original Linked List: ")
print_list(head)
head = del_head(head)
print("Updated Linked List: ")
print_list(head)
Output:
Original Linked List:
1 2 3
Updated Linked List:
2 3
Time Complexity: O(1), Since traversal of the linked list is not required.
Auxiliary Space: O(1)
Example Execution
Initial Linked List:
1 <-> 2 <-> 3
- Head: Points to the first node with data
1
. - Prev of Head:
NULL
(since it’s the first node). - Next of Head: Points to the node with data
2
.
After Deleting the Head Node:
2 <-> 3
- Head: Now points to the second node with data
2
. - Prev of New Head: Updated to
NULL
. - Memory for Old Head (1): Freed.
Analysis
Time Complexity
The time complexity of this operation is O(1). This is because no traversal of the list is required. All updates involve direct pointer manipulations, which are executed in constant time.
Space Complexity
The auxiliary space complexity is O(1) since no additional data structures are used, and only a temporary pointer is needed for managing the deletion.
Key Considerations
- Boundary Cases:
- If the list is empty, ensure the function returns
NULL
without any errors. - Deleting the head of a single-node list results in an empty list, so the
head
must be updated toNULL
.
- If the list is empty, ensure the function returns
- Memory Management:
Always free memory is allocated for the deleted node to avoid memory leaks, which are critical in C programming. - Pointer Integrity:
Carefully update bothprev
andnext
pointers to avoid dangling references and undefined behavior.
Applications
The ability to efficiently delete nodes at the beginning is useful in numerous scenarios, such as:
- Implementing queues or stacks using linked lists.
- Optimizing memory usage in real-time systems.
- Managing dynamic data structures where elements are frequently added or removed.
Advantages of Deletion at the Beginning in a Doubly Linked List
The operation of deleting a node at the beginning of a doubly linked list comes with several advantages due to the unique properties of the data structure. These advantages make it an efficient and preferred choice for certain real-world applications. Below are the key benefits:
- Constant Time Complexity {O(1)}
- Deleting a node at the beginning of a doubly linked list is highly efficient with a time complexity of O(1). Unlike other operations that might require traversing the list (e.g., deleting a node at a specific position), this operation only involves:
- Updating the head pointer.
- Setting the
prev
pointer of the new head toNULL
. - Freeing the old node.
- This constant-time operation is particularly beneficial in applications where performance is critical.
- No Need for Traversal
- Unlike deleting a node from the middle or end of the list, deleting the head node does not require traversing the list. Since the head pointer is already accessible, the operation focuses solely on pointer updates, making it straightforward and efficient.
- Maintains Structural Integrity
- The bidirectional nature of a doubly linked list ensures that after deleting the head node, the remaining list remains intact. By properly updating the
prev
pointer of the new head, the structural integrity of the list is preserved, allowing for seamless subsequent operations like insertions or deletions.
- The bidirectional nature of a doubly linked list ensures that after deleting the head node, the remaining list remains intact. By properly updating the
- Simplifies Dynamic Memory Management
- In languages like C or C++, memory must be manually managed. Deleting the head node involves freeing the memory allocated for the deleted node, preventing memory leaks. Since the head node is directly accessible, managing its memory is simpler compared to nodes deeper in the list.
- Ideal for Queue Implementation
- Queues operate on a First-In-First-Out (FIFO) basis, where elements are inserted at the rear and removed from the front. Deleting the head node in a doubly linked list aligns perfectly with this requirement, allowing efficient dequeuing without traversing the list.
- Useful for Real-Time Systems
- In real-time systems where responsiveness is crucial, the O(1)O(1) time complexity of head deletion ensures minimal delay. This is particularly advantageous in applications like:
- Task scheduling in operating systems.
- Packet processing in network routers.
- Bidirectional Traversal Not Required
- While a doubly linked list supports bidirectional traversal, deleting the head node only requires updates to the
next
andprev
pointers of the affected nodes. This localized operation minimizes computational overhead.
- While a doubly linked list supports bidirectional traversal, deleting the head node only requires updates to the
- Simplifies List Resetting
- When resetting a doubly linked list, nodes can be deleted one by one starting from the head. The ability to delete the head efficiently simplifies the process of clearing the entire list.
- Supports Applications with High Insert/Delete Frequency
- In applications where nodes are frequently added to and removed from the front of the list (e.g., maintaining a history stack or priority queue), the efficiency of deleting the head node enhances overall system performance.
- Foundation for More Complex Algorithms
- Mastering head deletion forms the basis for implementing more advanced data structures and algorithms. For example:
- Deque Operations: Deques rely on fast insertions and deletions at both ends.
- LRU Cache: Least Recently Used (LRU) caches often use a doubly linked list for efficient element removal and reordering.
Conclusion
Deleting the head node of a doubly linked list is a fundamental operation that demonstrates the versatility and efficiency of pointer-based data structures. By carefully updating the head pointer, setting the prev
pointer of the new head to NULL
, and freeing memory for the old head, this operation maintains the list’s structural integrity with a time complexity of O(1). Such efficiency highlights the strength of doubly linked lists in scenarios requiring frequent insertions and deletions.
Mastering this operation is crucial for developers working on real-time systems, memory-sensitive applications, or custom data structures. Understanding and implementing these techniques paves the way for building more complex and high-performing algorithms, reinforcing the importance of mastering core linked list manipulations in computer science.
Related Articles
- Deletion in a Doubly Linked List with Implementation: A Comprehensive Guide
- Deletion at the Beginning in a Doubly Linked List: A Detailed Exploration
- Deletion after a given node in Doubly Linked List: A Comprehensive Guide
- Deleting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Deletion at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Deletion at the End in Doubly Linked List: A Comprehensive Exploration
Read More Articles
- Data Structure (DS) Array:
- Why the Analysis of Algorithms is Important?
- Worst, Average, and Best Case Analysis of Algorithms: A Comprehensive Guide
- Understanding Pointers in C Programming: A Comprehensive Guide
- Understanding Arrays in Data Structures: A Comprehensive Exploration
- Memory Allocation of an Array: An In-Depth Comprehensive Exploration
- Understanding Basic Operations in Arrays: A Comprehensive Guide
- Understanding 2D Arrays in Programming: A Comprehensive Guide
- Mapping a 2D Array to a 1D Array: A Comprehensive Exploration
- Data Structure Linked List:
- Understanding Linked Lists in Data Structures: A Comprehensive Exploration
- Types of Linked List: Detailed Exploration, Representations, and Implementations
- Understanding Singly Linked Lists: A Detailed Exploration
- Understanding Doubly Linked List: A Comprehensive Guide
- Operations of Doubly Linked List with Implementation: A Detailed Exploration
- Insertion in Doubly Linked List with Implementation: A Detailed Exploration
- Inserting a Node at the Beginning of a Doubly Linked List: A Detailed Exploration
- Inserting a Node After a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Inserting a New Node at the End of a Doubly Linked List: A Detailed Exploration
- Deletion in a Doubly Linked List with Implementation: A Comprehensive Guide
- Deletion at the Beginning in a Doubly Linked List: A Detailed Exploration
- Deletion after a given node in Doubly Linked List: A Comprehensive Guide
- Deleting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Deletion at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Deletion at the End in Doubly Linked List: A Comprehensive Exploration
Frequently Asked Questions (FAQs)
What is a doubly linked list, and how does it differ from a singly linked list?
A doubly linked list is a linear data structure in which each node contains three fields:
- Data: Holds the value of the node.
- Next Pointer: Points to the next node in the sequence.
- Previous Pointer: Points to the previous node in the sequence.
This structure allows bidirectional traversal, making certain operations, like deletion and insertion, more efficient than in a singly linked list, where each node has only a next
pointer. However, the trade-off is the additional memory overhead for the prev
pointer and slightly more complex pointer manipulation.
Why is it important to update the previous pointer of the new head node when deleting the old head?
The previous pointer (prev) of the new head node must be set to NULL
after the old head is deleted to ensure the structural integrity of the doubly linked list. If this step is missed, the new head node may still reference the deleted node, creating a dangling pointer. This can lead to undefined behavior, such as accessing invalid memory locations, causing the program to crash.
What are the key steps involved in deleting the head node of a doubly linked list?
To delete the head node in a doubly linked list, follow these steps:
- Check if the list is empty: If
head == NULL
, there is nothing to delete, so returnNULL
. - Store the head node in a temporary variable: This ensures the node can be safely deallocated later without losing access to the rest of the list.
- Update the head pointer: Move the
head
pointer to the next node (head = head->next
). - Update the new head’s
prev
pointer: Set theprev
pointer of the new head node toNULL
if it exists. - Free the old head node: Use the
free()
function to release the memory allocated for the old head. - Return the new head pointer: Return the updated head pointer for further use.
How is memory managed when deleting a node in C?
In C programming, memory for dynamically allocated nodes is manually managed using the malloc()
and free()
functions. When a node is deleted, the memory allocated for it must be released using free(temp)
(where temp
is the pointer to the node being deleted). If memory is not freed, it can cause a memory leak, leading to inefficient memory utilization, especially in long-running programs.
What is the time complexity of deleting the head node, and why?
The time complexity of deleting the head node in a doubly linked list is O(1)O(1). This is because the operation involves a constant number of pointer updates:
- Updating the
head
pointer to the next node. - Updating the
prev
pointer of the new head node. - Freeing the old head node.
Since no traversal of the list is required, the time complexity remains constant regardless of the list’s length.
How does the deletion process handle an empty list or a single-node list?
- Empty List: If the list is empty (
head == NULL
), the function returnsNULL
, as there is no node to delete. - Single-Node List: If the list contains only one node, updating the
head
pointer toNULL
effectively makes the list empty. The memory for the lone node is then freed, and the function returnsNULL
.
Why is freeing memory essential, and what are the risks of not doing so?
Freeing memory is essential in C programming to prevent memory leaks. A memory leak occurs when dynamically allocated memory is no longer accessible (e.g., a pointer to it is deleted or overwritten), but the memory is not deallocated. Over time, this can lead to excessive memory usage, causing the program or system to crash due to insufficient memory. By freeing the old head node after deletion, we ensure efficient memory usage and program stability.
Can this operation be performed in other programming languages like Python or Java?
Yes, the concept of deleting the head node applies to other programming languages, but the implementation details differ:
- Python: Python uses automatic memory management (garbage collection). When the reference to the old head node is removed, Python automatically reclaims its memory.
- Java: Similar to Python, Java relies on garbage collection. Once there are no references to the old head node, its memory is automatically freed.
In contrast, C and C++ require explicit memory management using free()
or delete
.
What happens if we forget to set the previous pointer of the new head to NULL?
If the prev
pointer of the new head is not set to NULL
, it will still reference the deleted node. This creates a dangling pointer, which can lead to serious issues such as:
- Accessing invalid memory, resulting in segmentation faults or undefined behavior.
- Compromising the integrity of the doubly linked list, making further operations unreliable.
Proper pointer management is crucial in maintaining the structure and reliability of the list.
What are the real-world applications of doubly linked lists, and why is efficient deletion important?
Doubly linked lists are widely used in real-world applications where efficient insertion and deletion are critical. Examples include:
- Implementing Deques: Doubly linked lists allow insertion and deletion from both ends in constant time, making them ideal for implementing queues and stacks.
- Navigating Browsers: Web browsers use doubly linked lists to maintain a history stack, enabling forward and backward navigation.
- Memory Management: Operating systems use doubly linked lists to track free and allocated memory blocks.
- Undo/Redo Operations: In text editors or spreadsheets, doubly linked lists efficiently manage undo and redo functionality.
Efficient deletion, such as removing the head node in O(1), enhances the performance of these systems, ensuring smooth and responsive user experiences.