Doubly Linked Lists (DLLs) are a fundamental data structure in computer science, frequently used in scenarios that demand dynamic memory management and efficient node traversal. One of the most common operations on a Doubly Linked List is insertion, where a new node is added at a specific position. In this article, we will focus on the process of inserting a new node after a specified node in a Doubly Linked List.
We will explore the detailed approach to performing this operation, including its implementation, the steps involved, and edge cases to consider. Let’s begin by understanding the context of the problem through examples.
Table of Contents
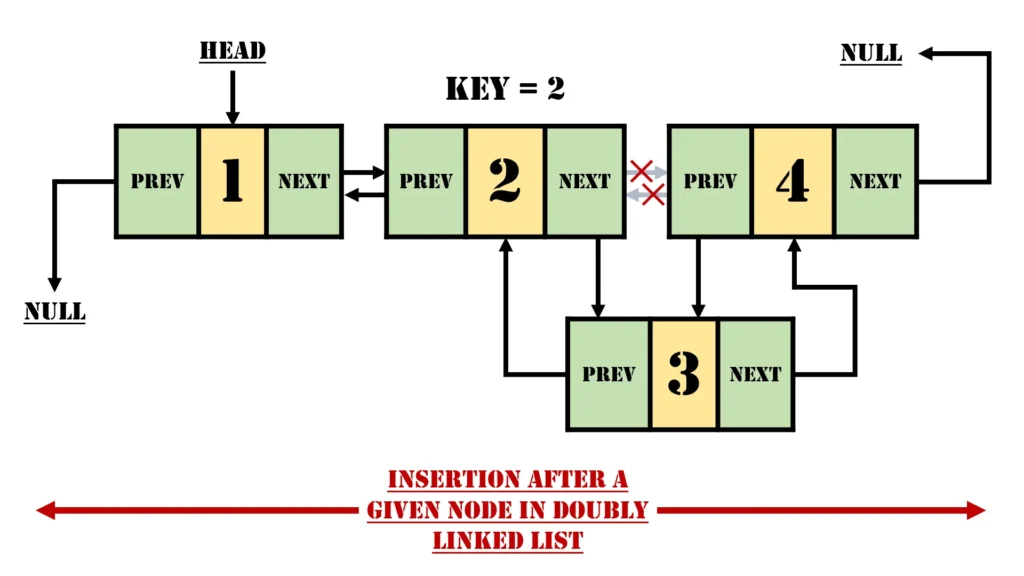
Understanding the Problem
The goal is to insert a new node with specified data into a Doubly Linked List after a given node.
Example 1
Input:
Linked List = 1 <-> 2 <-> 4
newData = 3
key = 2
Output:
Linked List = 1 <-> 2 <-> 3 <-> 4
Explanation:
The new node with value 3
is inserted after the node with value 2
. The Doubly Linked List is updated to reflect this change.
Example 2
Input:
Linked List = 1 <-> 2
newData = 4
key = 2
Output:
Linked List = 1 <-> 2 <-> 4
Explanation:
The new node with value 4
is inserted after the node with value 2
. Since this is the last node in the list, no further adjustments to pointers are necessary.
Detailed Approach
To solve this problem, a structured approach is required. Let’s break it down into logical steps:
1. Traversing the Doubly Linked List
Before inserting a node, we need to locate the target node where the insertion will occur. This is done by traversing the Doubly Linked List.
- Start at the head of the list.
- Compare the value of each node to the given key.
- If the node with the key is found, store a reference to it and proceed to the next step.
- If the key does not exist, return the original list without any modifications.
2. Creating a New Node
Once the target node is found, create a new node using the provided data.
- Allocate memory dynamically for the new node (in languages like C/C++) or instantiate it using the appropriate syntax (e.g.,
new Node()
in Java or Python classes). - Set the data of the new node to the given value (
newData
).
3. Updating the Pointers
Updating the pointers is the most crucial part of this operation. In a Doubly Linked List, each node contains two pointers:
- prev: Points to the previous node.
- next: Points to the next node.
To correctly insert the new node, follow these steps:
- Set the
prev
pointer of the new node to the target node (curr
). - Set the
next
pointer of the new node to thenext
pointer of the target node. - Update the
next
pointer of the target node to point to the new node.
4. Handling Edge Cases
If the new node is being inserted at the end of the list, the next
pointer of the new node will be NULL
. Otherwise:
- Update the
prev
pointer of the node that comes after the new node to point to the new node.
By handling these cases, we ensure that the list remains intact and traversal in both directions continues to function correctly.
Algorithm
Below is the step-by-step algorithm for inserting a node after a given node in a Doubly Linked List:
- Traverse the list to find the node with the specified key (
curr
). - If the key is not found, return the original list.
- Create a new node (
new_node
) with the given data. - Update the pointers:
new_node->prev = curr
new_node->next = curr->next
curr->next = new_node
- If the new node is not the last node, update:
new_node->next->prev = new_node
Implementation In Python
Let’s translate this logic into Python code:
class Node:
def __init__(self, data):
self.data = data
self.prev = None
self.next = None
class DoublyLinkedList:
def __init__(self):
self.head = None
def insert_after(self, key, new_data):
# Traverse the list to find the target node
curr = self.head
while curr and curr.data != key:
curr = curr.next
# If key is not found, return without changes
if not curr:
print(f"Node with value {key} not found.")
return
# Create the new node
new_node = Node(new_data)
# Update pointers
new_node.prev = curr
new_node.next = curr.next
curr.next = new_node
if new_node.next:
new_node.next.prev = new_node
def display(self):
curr = self.head
while curr:
print(curr.data, end=" <-> " if curr.next else "")
curr = curr.next
print()
# Example Usage
dll = DoublyLinkedList()
dll.head = Node(1)
node2 = Node(2)
node4 = Node(4)
dll.head.next = node2
node2.prev = dll.head
node2.next = node4
node4.prev = node2
print("Original List:")
dll.display()
dll.insert_after(2, 3)
print("After Insertion:")
dll.display()
Implementation In Multiple Programming Languages
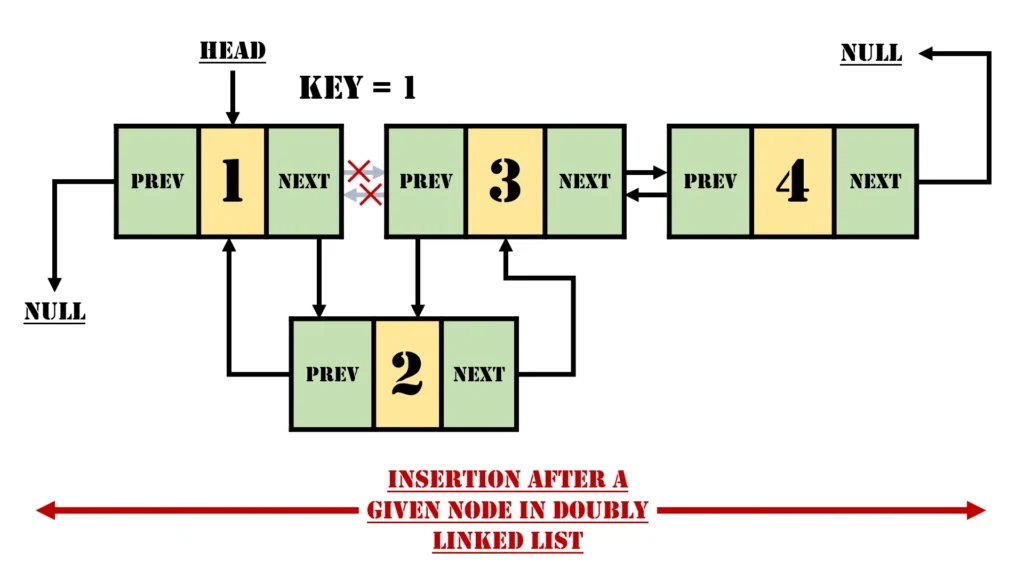
Here is the code written in C, C++, C#, Java, JavaScript, and Python, along with a detailed step-by-step explanation for each language.
C Implementation
#include <stdio.h>
#include <stdlib.h>
// Define the structure of a doubly linked list node
struct Node {
int data;
struct Node *next;
struct Node *prev;
};
// Function to create a new node with the given data
struct Node *createNode(int new_data) {
struct Node *new_node = (struct Node *)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = NULL;
new_node->prev = NULL;
return new_node;
}
// Function to insert a new node after a given node
struct Node *insertAfter(struct Node *head, int key, int new_data) {
struct Node *curr = head;
// Traverse the list to find the node with the given key
while (curr != NULL) {
if (curr->data == key)
break;
curr = curr->next;
}
// If key is not found, return the original head
if (curr == NULL)
return head;
// Create a new node
struct Node *new_node = createNode(new_data);
// Update links to insert the new node
new_node->prev = curr;
new_node->next = curr->next;
// Update the next pointer of the current node
curr->next = new_node;
// Update the previous pointer of the new node's next
if (new_node->next != NULL)
new_node->next->prev = new_node;
return head;
}
// Function to print the doubly linked list
void printList(struct Node *head) {
struct Node *curr = head;
while (curr != NULL) {
printf(" %d", curr->data);
curr = curr->next;
}
printf("\n");
}
int main() {
// Create a doubly linked list: 1 <-> 3 <-> 4
struct Node *head = createNode(1);
head->next = createNode(3);
head->next->prev = head;
head->next->next = createNode(4);
head->next->next->prev = head->next;
// Print the original list
printf("Original Linked List:");
printList(head);
// Insert a new node after node with key 1
printf("Inserting Node with data 2 after node 1 :");
head = insertAfter(head, 1, 2);
// Print the updated list
printList(head);
return 0;
}
Step by Step Explanation
- Structure Definition:
- The
Node
structure has three fields:data
: Stores the value.next
: Points to the next node in the list.prev
: Points to the previous node.
- The
createNode
Function:- Allocates memory for a new node.
- Initializes
data
,next
, andprev
.
insertAfter
Function:- Traverses the list to find the node with the given key.
- If the key is found, creates a new node and updates:
prev
of the new node to point to the current node.next
of the new node to point to the current node’s next.
- Updates the
next
pointer of the current node to point to the new node. - Updates the
prev
pointer of the next node (if it exists) to point back to the new node.
- Printing Function:
- Traverses the list and prints
data
of each node.
- Traverses the list and prints
- Main Function:
- Creates a doubly linked list:
1 <-> 3 <-> 4
. - Inserts
2
after1
. - Prints both the original and updated lists.
- Creates a doubly linked list:
C++ Implementation
#include <iostream>
using namespace std;
struct Node {
int data;
Node* next;
Node* prev;
Node(int new_data) : data(new_data), next(nullptr), prev(nullptr) {}
};
// Function to insert a node after a given node
Node* insertAfter(Node* head, int key, int new_data) {
Node* curr = head;
// Traverse to find the key
while (curr) {
if (curr->data == key)
break;
curr = curr->next;
}
// If key is not found, return the original head
if (!curr)
return head;
// Create new node and update links
Node* new_node = new Node(new_data);
new_node->prev = curr;
new_node->next = curr->next;
curr->next = new_node;
if (new_node->next)
new_node->next->prev = new_node;
return head;
}
// Function to print the list
void printList(Node* head) {
Node* curr = head;
while (curr) {
cout << " " << curr->data;
curr = curr->next;
}
cout << endl;
}
int main() {
// Create a doubly linked list: 1 <-> 3 <-> 4
Node* head = new Node(1);
head->next = new Node(3);
head->next->prev = head;
head->next->next = new Node(4);
head->next->next->prev = head->next;
// Print the original list
cout << "Original Linked List:";
printList(head);
// Insert a new node after node with key 1
cout << "Inserting Node with data 2 after node 1 :";
head = insertAfter(head, 1, 2);
// Print the updated list
printList(head);
return 0;
}
Step by Step Explanation
- Structure Definition:
- The
Node
structure has three fields:data
: Stores the value.next
: Points to the next node in the list.prev
: Points to the previous node.
- The
createNode
Function:- Allocates memory for a new node.
- Initializes
data
,next
, andprev
.
insertAfter
Function:- Traverses the list to find the node with the given key.
- If the key is found, creates a new node and updates:
prev
of the new node to point to the current node.next
of the new node to point to the current node’s next.
- Updates the
next
pointer of the current node to point to the new node. - Updates the
prev
pointer of the next node (if it exists) to point back to the new node.
- Printing Function:
- Traverses the list and prints
data
of each node.
- Traverses the list and prints
- Main Function:
- Creates a doubly linked list:
1 <-> 3 <-> 4
. - Inserts
2
after1
. - Prints both the original and updated lists.
- Creates a doubly linked list:
C# Implementation
using System;
class Node {
public int data;
public Node next;
public Node prev;
public Node(int data) {
this.data = data;
this.next = null;
this.prev = null;
}
}
class DoublyLinkedList {
public Node InsertAfter(Node head, int key, int newData) {
Node current = head;
// Traverse the list to find the key
while (current != null) {
if (current.data == key)
break;
current = current.next;
}
// If key is not found, return the original head
if (current == null) return head;
// Create a new node
Node newNode = new Node(newData);
// Update links to insert the new node
newNode.prev = current;
newNode.next = current.next;
if (current.next != null)
current.next.prev = newNode;
current.next = newNode;
return head;
}
public void PrintList(Node head) {
Node current = head;
while (current != null) {
Console.Write(" " + current.data);
current = current.next;
}
Console.WriteLine();
}
}
class Program {
static void Main(string[] args) {
DoublyLinkedList dll = new DoublyLinkedList();
// Create a doubly linked list: 1 <-> 3 <-> 4
Node head = new Node(1);
head.next = new Node(3);
head.next.prev = head;
head.next.next = new Node(4);
head.next.next.prev = head.next;
// Print the original list
Console.WriteLine("Original Linked List:");
dll.PrintList(head);
// Insert a new node after node with key 1
Console.WriteLine("Inserting Node with data 2 after node 1 :");
head = dll.InsertAfter(head, 1, 2);
// Print the updated list
dll.PrintList(head);
}
}
Explanation
- Class
Node
:- Defines a doubly linked list node with
data
,next
, andprev
properties.
- Defines a doubly linked list node with
- Class
DoublyLinkedList
:- Contains the method
InsertAfter
to insert a new node after a specific key. - Updates the links (
next
andprev
) of the nodes involved.
- Contains the method
InsertAfter
Method:- Traverses the list to find the key.
- If the key is found:
- Creates a new node.
- Updates the pointers of the current node and the new node.
- Updates the
prev
pointer of the new node’s next node, if it exists.
- Printing Method:
- Traverses the list from the head, printing each node’s
data
.
- Traverses the list from the head, printing each node’s
Main
Method:- Creates a doubly linked list with hardcoded values.
- Inserts a node with
data = 2
afterdata = 1
. - Prints the original and updated lists.
Java Implementation
class Node {
int data;
Node next;
Node prev;
Node(int data) {
this.data = data;
this.next = null;
this.prev = null;
}
}
class DoublyLinkedList {
// Method to insert a new node after a given key
Node insertAfter(Node head, int key, int newData) {
Node current = head;
// Traverse to find the key
while (current != null) {
if (current.data == key)
break;
current = current.next;
}
// If key is not found, return the original head
if (current == null) return head;
// Create a new node
Node newNode = new Node(newData);
// Update links
newNode.prev = current;
newNode.next = current.next;
if (current.next != null)
current.next.prev = newNode;
current.next = newNode;
return head;
}
// Method to print the list
void printList(Node head) {
Node current = head;
while (current != null) {
System.out.print(" " + current.data);
current = current.next;
}
System.out.println();
}
}
public class Main {
public static void main(String[] args) {
DoublyLinkedList dll = new DoublyLinkedList();
// Create a doubly linked list: 1 <-> 3 <-> 4
Node head = new Node(1);
head.next = new Node(3);
head.next.prev = head;
head.next.next = new Node(4);
head.next.next.prev = head.next;
// Print the original list
System.out.println("Original Linked List:");
dll.printList(head);
// Insert a new node after node with key 1
System.out.println("Inserting Node with data 2 after node 1 :");
head = dll.insertAfter(head, 1, 2);
// Print the updated list
dll.printList(head);
}
}
Step-by-Step Explanation
1. Node
Class
The Node
class represents each node in the doubly linked list.
- Attributes:
data
: Stores the integer data for the node.next
: Points to the next node in the list.prev
: Points to the previous node in the list.
- Constructor:
- Initializes the
data
attribute with the given value. - Sets
next
andprev
pointers tonull
.
- Initializes the
2. DoublyLinkedList
Class
(a.) insertAfter
Method
- Input Parameters:
Node head
: The head node of the doubly linked list.int key
: The value after which the new node will be inserted.int newData
: The data for the new node.
- Traversal:
- Starts traversing the list from the
head
using a pointer (current
). - Compares the
data
of each node withkey
until it finds the node with thekey
or reaches the end of the list.
- Starts traversing the list from the
- If
key
Is Not Found:- If the traversal completes without finding the key (
current == null
), the method returns the original list without making any changes.
- If the traversal completes without finding the key (
- Node Creation:
- Creates a new
Node
object, passingnewData
to its constructor.
- Creates a new
- Updating Links:
- Sets
newNode.prev = current
(points the new node’sprev
to the node containing thekey
). - Sets
newNode.next = current.next
(points the new node’snext
to the node that follows thecurrent
node). - If
current.next
exists, updates itsprev
pointer to thenewNode
. - Updates
current.next
to point tonewNode
, effectively inserting the new node after thecurrent
node.
- Sets
(b.) printList
Method
- Traversal:
- Starts at the
head
node and iterates through the list. - Prints the
data
of each node until the end of the list is reached.
- Starts at the
3. Main
Class
- Creating the Doubly Linked List:
- Constructs a hardcoded list:
1 <-> 3 <-> 4
. - Manually links the
next
andprev
pointers for each node.
- Constructs a hardcoded list:
- Printing the Original List:
- Calls the
printList
method to display the initial list.
- Calls the
- Inserting a New Node:
- Calls the
insertAfter
method to insert a node withdata = 2
after the node withdata = 1
.
- Calls the
- Printing the Updated List:
- Calls the
printList
method to display the modified list.
- Calls the
JavaScript Implementation
class Node {
constructor(data) {
this.data = data;
this.next = null;
this.prev = null;
}
}
class DoublyLinkedList {
insertAfter(head, key, newData) {
let current = head;
// Traverse to find the key
while (current) {
if (current.data === key) break;
current = current.next;
}
// If key is not found, return the original head
if (!current) return head;
// Create a new node
const newNode = new Node(newData);
// Update links
newNode.prev = current;
newNode.next = current.next;
if (current.next) current.next.prev = newNode;
current.next = newNode;
return head;
}
printList(head) {
let current = head;
let result = [];
while (current) {
result.push(current.data);
current = current.next;
}
console.log(result.join(" "));
}
}
// Create a doubly linked list: 1 <-> 3 <-> 4
const dll = new DoublyLinkedList();
let head = new Node(1);
head.next = new Node(3);
head.next.prev = head;
head.next.next = new Node(4);
head.next.next.prev = head.next;
// Print the original list
console.log("Original Linked List:");
dll.printList(head);
// Insert a new node after node with key 1
console.log("Inserting Node with data 2 after node 1 :");
head = dll.insertAfter(head, 1, 2);
// Print the updated list
dll.printList(head);
Step-by-Step Explanation
1. Node
Class
The Node
class represents a node in the doubly linked list.
- Attributes:
data
: Stores the value of the node.next
: Points to the next node in the list.prev
: Points to the previous node in the list.
- Constructor:
- Accepts the
data
and initializes thenext
andprev
pointers asnull
.
- Accepts the
2. DoublyLinkedList
Class
(a.) insertAfter
Method
- Input Parameters:
head
: The head node of the doubly linked list.key
: The value after which the new node will be inserted.newData
: The data for the new node.
- Traversal:
- Uses a variable
current
to traverse the list from thehead
. - Checks if the
data
of each node matches thekey
.
- Uses a variable
- If
key
Is Not Found:- If the traversal completes and the
key
is not found (current === null
), the method returns the original list unchanged.
- If the traversal completes and the
- Node Creation:
- Creates a new
Node
object, passingnewData
to its constructor.
- Creates a new
- Updating Links:
- Sets
newNode.prev = current
(points the new node’sprev
to the node containing thekey
). - Sets
newNode.next = current.next
(points the new node’snext
to the node following thecurrent
node). - If
current.next
exists, updates itsprev
pointer to thenewNode
. - Updates
current.next
to point tonewNode
, inserting the new node after thecurrent
node.
- Sets
b. printList
Method
- Traversal:
- Starts from the
head
and iterates through the list. - Stores the
data
of each node in an array. - Joins the array into a string and prints the result.
- Starts from the
3. Main Program
- Creating the Doubly Linked List:
- Constructs a list:
1 <-> 3 <-> 4
. - Manually sets the
next
andprev
pointers for each node.
- Constructs a list:
- Printing the Original List:
- Calls the
printList
method to display the initial list.
- Calls the
- Inserting a New Node:
- Calls the
insertAfter
method to insert a node withdata = 2
after the node withdata = 1
.
- Calls the
- Printing the Updated List:
- Calls the
printList
method to display the modified list.
- Calls the
Python Implementation
class Node:
def __init__(self, data):
self.data = data
self.next = None
self.prev = None
class DoublyLinkedList:
# Function to insert a new node after a given key
def insert_after(self, head, key, new_data):
current = head
# Traverse to find the key
while current:
if current.data == key:
break
current = current.next
# If key is not found, return the original head
if not current:
return head
# Create a new node
new_node = Node(new_data)
# Update links
new_node.prev = current
new_node.next = current.next
if current.next:
current.next.prev = new_node
current.next = new_node
return head
# Function to print the list
def print_list(self, head):
current = head
while current:
print(current.data, end=" ")
current = current.next
print()
# Create a doubly linked list: 1 <-> 3 <-> 4
dll = DoublyLinkedList()
head = Node(1)
head.next = Node(3)
head.next.prev = head
head.next.next = Node(4)
head.next.next.prev = head.next
# Print the original list
print("Original Linked List:")
dll.print_list(head)
# Insert a new node after node with key 1
print("Inserting Node with data 2 after node 1:")
head = dll.insert_after(head, 1, 2)
# Print the updated list
dll.print_list(head)
Step-by-Step Explanation
1. Node Class
- Purpose: Represents each node in the doubly linked list.
- Attributes:
data
: Stores the data for the node.next
: Points to the next node in the list.prev
: Points to the previous node in the list.
- Initialization: The
__init__
constructor initializes thedata
,next
, andprev
pointers toNone
.
2. DoublyLinkedList Class
- This class contains utility methods for the doubly linked list.
(a.) insert_after
Method
- Input Parameters:
head
: The head node of the doubly linked list.key
: The value after which the new node is to be inserted.new_data
: The data of the new node.
- Traversal:
- Starts from the
head
and traverses through the list to locate the node containing thekey
. - If the
key
is not found (current
becomesNone
), it returns the original list without any modification.
- Starts from the
- Node Creation:
- A new node is created with
new_data
.
- A new node is created with
- Updating Links:
new_node.prev
is set tocurrent
(the node containing the key).new_node.next
is set tocurrent.next
.- If
current.next
exists, itsprev
is updated to point tonew_node
. - Finally,
current.next
is updated to point tonew_node
.
(b.) print_list
Method
- Traverses the list starting from the
head
and prints thedata
of each node until the end of the list.
3. Main Program
- List Creation:
- Creates a hardcoded doubly linked list:
1 <-> 3 <-> 4
. - Links are established manually for the
next
andprev
pointers of the nodes.
- Creates a hardcoded doubly linked list:
- Print the Original List:
- Calls the
print_list
method to display the list.
- Calls the
- Insert New Node:
- Calls the
insert_after
method to insert a node withdata = 2
after the node withdata = 1
.
- Calls the
- Print the Updated List:
- Prints the modified list to confirm the insertion.
Output
Original Linked List:
1 3 4
Inserting Node with data 2 after node 1:
1 2 3 4
Time Complexity: O(n), where n is the number of nodes in the doubly linked list
Auxiliary Space: O(1)
Key Points to Remember
- Pointer Updates Are Crucial: A single incorrect pointer update can break the structure of the list, making it unusable.
- Edge Cases: Always handle cases where the insertion occurs at the end or the beginning of the list.
- Traversal Complexity: The time complexity for traversal is O(n), where
n
is the number of nodes in the list.
Advantages of Doubly Linked Lists
- Bidirectional Traversal: The presence of two pointers in each node allows for traversal in both directions.
- Dynamic Size: Doubly Linked Lists can dynamically grow or shrink as needed without requiring memory reallocation.
- Efficient Insertions/Deletions: Unlike arrays, DLLs allow for efficient insertion and deletion at any position.
Key Differences Between Insertion Before and After a Node in a Doubly Linked List
The operations of inserting a node before and after a specific node in a Doubly Linked List (DLL) differ in terms of pointer updates and the positioning of the new node relative to the target node.
Aspect | Insertion Before a Node | Insertion After a Node |
---|---|---|
Definition | The new node is inserted before a specified target node in the Doubly Linked List (DLL). | The new node is inserted after a specified target node in the Doubly Linked List (DLL). |
Pointer Updates | – new_node->next = curr (new node points to target node).– new_node->prev = curr->prev (new node points to target’s previous node).– If curr->prev != NULL , set curr->prev->next = new_node .– curr->prev = new_node . | – new_node->prev = curr (new node points to target node).– new_node->next = curr->next (new node points to target’s next node).– If curr->next != NULL , set curr->next->prev = new_node .– curr->next = new_node . |
Target Node Role | The target node becomes the next node of the newly inserted node. | The target node becomes the previous node of the newly inserted node. |
Special Cases | – If the target node is the head, the new node becomes the new head, and the list’s head pointer must be updated. | – If the target node is the tail, the new node becomes the new tail, and its next pointer is set to NULL . |
Edge Cases | – Target node is the head of the list. – List is empty. – Key not found. | – Target node is the tail of the list. – List is empty. – Key not found. |
Memory Allocation | Memory is allocated for the new node, and appropriate pointers are adjusted to maintain the structure of the list. | Similar memory allocation is done, but the pointer adjustments differ based on the relative position of the new node. |
Example (Original List) | 1 <-> 2 <-> 4 | 1 <-> 2 <-> 4 |
Example Operation | Insert 3 before node with key 4 . | Insert 3 after node with key 2 . |
Resulting List | 1 <-> 2 <-> 3 <-> 4 | 1 <-> 2 <-> 3 <-> 4 |
Complexity | – Traversal: O(n), as the target node must be located. – Insertion: O(1) once the target is found. | – Traversal: O(n), as the target node must be located. – Insertion: O(1) once the target is found. |
When to Use | Use when the new node must appear before the specified node, such as adding a node at the head or maintaining an ordered list in ascending order. | Use when the new node must appear after the specified node, such as appending a node at the tail or adding an intermediate node in a sequence. |
Key Takeaways
- Pointer Adjustments Differ: The main difference lies in how the
prev
andnext
pointers of the involved nodes are updated. - Edge Case Handling: Both operations require careful handling of edge cases, such as when the target node is the head, tail, or in an empty list.
- Efficiency: Both operations have the same time complexity, with O(n) for traversal and O(1) for pointer updates.
- Choice of Operation: The decision to insert before or after depends entirely on the desired position of the new node relative to the target node.
FAQs: Inserting a Node After a Given Node in a Doubly Linked List
What is a Doubly Linked List (DLL)?
A Doubly Linked List (DLL) is a type of linked data structure in which each node contains three components:
- Data: The actual value stored in the node.
- Prev Pointer: A pointer to the previous node in the sequence.
- Next Pointer: A pointer to the next node in the sequence.
Unlike a Singly Linked List, a DLL allows bidirectional traversal, meaning you can navigate both forward and backward through the list. This makes certain operations, such as insertion and deletion, more efficient.
Why is it important to update both prev
and next
pointers in a DLL?
In a Doubly Linked List, each node maintains references to its neighboring nodes through its prev
and next
pointers. When inserting or deleting a node, failing to update these pointers can cause:
- Broken Links: The list may lose connectivity, making traversal impossible.
- Memory Leaks: Dangling pointers to deleted nodes could lead to unreferenced memory.
For example, during an insertion, the prev
pointer of the new node must point to the target node, and its next
pointer must link to the node that originally came after the target.
What are the steps to insert a node after a given node in a DLL?
To insert a node after a specific node in a DLL, follow these steps:
- Locate the Target Node: Traverse the list to find the node with the specified key (
curr
). - Create a New Node: Instantiate a new node (
new_node
) and assign the provided data to it. - Update Pointers:
- Set
new_node->prev = curr
. - Set
new_node->next = curr->next
. - Update
curr->next
to point tonew_node
. - If
new_node->next
is notNULL
, update itsprev
pointer to point tonew_node
.
- Set
These steps ensure that the new node is seamlessly integrated into the list.
How do you handle edge cases when inserting a node in a DLL?
Several edge cases need to be considered when inserting a node in a DLL:
- Empty List: If the list is empty, the new node becomes the head of the list.
- End of List: If the new node is inserted at the end, its
next
pointer will beNULL
, and only itsprev
pointer needs updating. - Middle of List: Both
prev
andnext
pointers of neighboring nodes must be updated correctly. - Key Not Found: If the specified key does not exist, return the list unchanged or display an error message.
What is the time complexity for inserting a node in a DLL?
The time complexity for inserting a node depends on the traversal to locate the target node.
- Best Case: O(1), if the target node is the head or a pointer to it is already provided.
- Worst Case: O(n), where
n
is the number of nodes, as you may need to traverse the entire list to find the target node.
Pointer updates during insertion are O(1) operations.
Can the same method be applied if the DLL is circular?
Yes, the insertion method can be adapted for Circular Doubly Linked Lists (CDLLs). In a CDLL:
- The last node’s
next
pointer points to the head. - The head’s
prev
pointer points to the last node.
When inserting a new node after a given node in a CDLL:
- Update the
prev
andnext
pointers of the new node as usual. - If the new node is inserted at the tail, ensure the circular connection is maintained by updating the head and tail references accordingly.
What are the advantages of using a DLL over a Singly Linked List?
A Doubly Linked List offers several advantages over a Singly Linked List:
- Bidirectional Traversal: You can move forward and backward through the list, which is not possible in a Singly Linked List.
- Efficient Insertions and Deletions: Insertions and deletions are faster in a DLL because you can access both neighboring nodes directly.
- Reversibility: Reversing a DLL is simpler since each node contains a
prev
pointer. - Flexibility: A DLL can handle more complex operations like adding nodes at specific indices efficiently.
How do you ensure the list remains consistent after inserting a node?
To maintain list consistency:
- Always update both
prev
andnext
pointers of all affected nodes. - Handle edge cases like insertion at the head, tail, or when the list is empty.
- Use proper memory allocation (in C/C++) or garbage collection (in languages like Python/Java) to manage memory.
Can a DLL be implemented in all programming languages?
Yes, Doubly Linked Lists can be implemented in virtually any programming language. The implementation syntax and memory management depend on the language:
- In Python, use classes to define nodes and manage pointers. Python’s built-in garbage collector simplifies memory management.
- In C/C++, manually allocate memory using
malloc
ornew
and free it when the node is no longer needed. - In Java, use classes and rely on its automatic garbage collection for memory management.
- In JavaScript, DLLs can be implemented using objects or ES6 classes.
What are the applications of Doubly Linked Lists?
Doubly Linked Lists are widely used in various real-world applications, such as:
- Browser History: Storing and navigating through the forward and backward history.
- Undo/Redo Functionality: Managing states in applications like text editors.
- Music Playlists: Enabling bidirectional navigation through songs.
- Memory Management: Implementing data structures like LRU Cache.
- Game Development: Storing objects like game states or player moves.
Mastering operations like inserting a node in a Doubly Linked List unlocks powerful tools for implementing efficient data structures, which are the backbone of modern computer science and software engineering.