Linked lists are a fundamental data structure in computer science, and understanding them is crucial for tackling complex data management problems efficiently. In this article, we’ll dive deep into the concept of linked lists, exploring their structure, benefits, and types, as well as how they differ from arrays and where they excel in various programming applications.
Table of Contents
What is a Linked List?
A linked list is a linear data structure comprising a sequence of nodes, where each node is connected to the next. Unlike arrays, which store elements contiguously in memory, linked lists are scattered in memory, with each node containing a data part and a reference (pointer) to the next node. The final node in a linked list contains a pointer to NULL
, marking the end of the list.
Representation of a Linked List
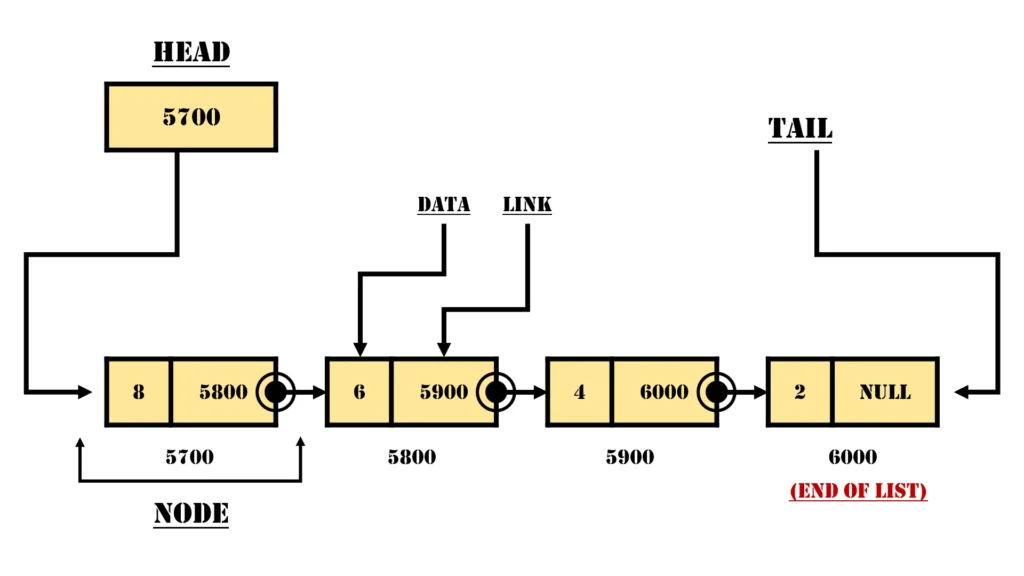
In a linked list, nodes are represented as individual elements connected by pointers. The structure of each node includes:
- Data Part – Stores the actual information the node represents.
- Address Part – Contains a pointer that links to the next node in the list.
For example, the linked list can be illustrated as follows:
Node1(data) --> Node2(data) --> Node3(data) --> NULL
Each node connects to the next node, and the last node points to NULL
, signifying the end of the list.
Why Use a Linked List Instead of an Array?
While arrays are a widely-used data structure, they come with some inherent limitations that linked lists can help to overcome:
- Fixed Size Requirement: Arrays have a predefined, fixed size that must be specified at the beginning of the program. Adjusting the size requires reallocating memory, which is time-consuming.
- Contiguous Memory Requirement: All array elements must occupy contiguous memory locations, limiting flexibility and making insertion and deletion inefficient.
- Inflexible Insertion/Deletion: Inserting or deleting elements in an array can be challenging since it may require shifting elements to maintain order.
Advantages of Linked Lists
Linked lists offer several advantages that address the limitations of arrays:
- Dynamic Memory Allocation: Linked lists can expand or shrink based on the program’s needs without wasting memory.
- Efficient Insertion and Deletion: Adding or removing elements in a linked list is easier, as only pointers need to be updated, not all elements.
- Memory Efficiency: Since they don’t require contiguous memory, linked lists can make use of available memory more efficiently.
Declaring a Linked List
Declaring a linked list differs from declaring arrays. Arrays are straightforward as they contain elements of the same type. However, a linked list contains two parts: the data part (of a specific data type) and a pointer part (which stores addresses).
In C programming, a linked list node can be declared using struct
:
struct node {
int data;
struct node *next;
};
In this example, struct node
is a user-defined data type that includes two fields: data
(an integer) and next
(a pointer to the next node).
Types of Linked Lists
There are various types of linked lists, each with unique characteristics and use cases:
1. Singly Linked List
A singly linked list is the most basic type, where each node points to the next node, forming a one-way chain. In a singly linked list, each node has two components: the data part (which stores information) and the link part (which points to the next node).
Example:
Node1(data) --> Node2(data) --> Node3(data) --> NULL
2. Doubly Linked List
A doubly linked list extends the functionality of a singly linked list by including a pointer to the previous node in addition to the pointer to the next node. This allows traversal in both forward and backward directions, making it more versatile.
Example:
NULL <-- Node1(data) <--> Node2(data) <--> Node3(data) --> NULL
3. Circular Singly Linked List
In a circular singly linked list, the last node doesn’t point to NULL
; instead, it points back to the first node, forming a circle. This configuration is ideal for applications requiring continuous looping.
Example:
Node1(data) --> Node2(data) --> Node3(data) --> Node1(data) ...
4. Circular Doubly Linked List
A circular doubly linked list is similar to a circular singly linked list but includes pointers to both the previous and next nodes, creating a fully bidirectional circular structure.
Example:
<-- Node1(data) <--> Node2(data) <--> Node3(data) <--> Node1(data) ...
Advantages of Using Linked Lists
- Dynamic Structure: The size of a linked list can grow or shrink according to the needs of the program.
- Efficient Insertion and Deletion: Unlike arrays, where elements are shifted, linked lists only require pointer adjustments.
- Memory Efficiency: Linked lists dynamically allocate memory, reducing waste.
- Implementation of Other Data Structures: Stacks, queues, and even trees can be implemented using linked lists.
Disadvantages of Linked Lists
Despite their advantages, linked lists have some drawbacks:
- Memory Usage: Each node requires additional memory for the pointer, which can lead to higher memory usage.
- Traversal Time: Linked lists require sequential traversal, making access slower compared to arrays.
- Reverse Traversing: Backtracking is more challenging in singly linked lists, requiring doubly linked lists for easier reverse traversal, which, in turn, increases memory usage.
Applications of Linked Lists
Linked lists have broad applications in computer science and software development:
- Polynomial Representation: Linked lists are ideal for representing polynomials due to their dynamic nature.
- Sparse Matrices: Linked lists efficiently store sparse matrices with fewer non-zero elements.
- Implementing Stacks and Queues: Stacks and queues are easily implemented using linked lists due to the flexible insertion and deletion.
- Graphs: Linked lists represent adjacency lists in graph theory, an efficient representation for sparse graphs.
Operations on Linked Lists
Here are some fundamental operations supported by linked lists:
- Insertion: Adding a new node at the beginning, end, or a specific position.
- Deletion: Removing a node from the list.
- Display: Printing the elements of the list.
- Search: Finding a node in the list by traversing through it.
Example Code for Linked List Operations
Let’s look at a simple example in C to insert elements at the beginning of a singly linked list:
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
void insertAtBeginning(struct node** head, int newData) {
struct node* newNode = (struct node*)malloc(sizeof(struct node));
newNode->data = newData;
newNode->next = *head;
*head = newNode;
}
void displayList(struct node *node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
}
int main() {
struct node* head = NULL;
insertAtBeginning(&head, 1);
insertAtBeginning(&head, 2);
insertAtBeginning(&head, 3);
printf("Linked List: ");
displayList(head);
return 0;
}
In this example, we dynamically allocate memory for new nodes and insert them at the beginning of the list, illustrating the flexibility and efficiency of linked lists in managing memory dynamically.
Example of Implementation of Linked List in Multiple Programming Language
Here’s how to implement the linked list insertion and display operations in various programming languages (C, C++, C#, Java, and Python), along with step-by-step explanations.
Code:
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
void insertAtBeginning(struct node** head, int newData) {
struct node* newNode = (struct node*)malloc(sizeof(struct node)); // Allocate memory for a new node
newNode->data = newData; // Assign data to the new node
newNode->next = *head; // Point new node’s next to the current head
*head = newNode; // Update head to point to the new node
}
void displayList(struct node *node) {
while (node != NULL) {
printf("%d ", node->data); // Display data of each node
node = node->next; // Move to the next node
}
}
int main() {
struct node* head = NULL;
insertAtBeginning(&head, 1); // Insert node with data 1
insertAtBeginning(&head, 2); // Insert node with data 2
insertAtBeginning(&head, 3); // Insert node with data 3
printf("Linked List: ");
displayList(head); // Display the list
return 0;
}
Explanation of C Code Steps:
- Define the Node Structure: We create a structure
node
with two parts:data
(integer) andnext
(pointer to the next node). - Insert at Beginning: This function creates a new node with the specified
newData
, points it to the current head, and updates the head to point to the new node. - Display List: This function traverses each node, printing its data until it reaches a
NULL
pointer. - Main Function: Initializes an empty linked list, inserts nodes at the beginning, and displays the final list.
Code:
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node* next;
Node(int data) {
this->data = data;
this->next = nullptr;
}
};
void insertAtBeginning(Node*& head, int newData) {
Node* newNode = new Node(newData); // Create a new node
newNode->next = head; // Point new node to current head
head = newNode; // Update head to new node
}
void displayList(Node* node) {
while (node != nullptr) {
cout << node->data << " ";
node = node->next;
}
}
int main() {
Node* head = nullptr;
insertAtBeginning(head, 1); // Insert node with data 1
insertAtBeginning(head, 2); // Insert node with data 2
insertAtBeginning(head, 3); // Insert node with data 3
cout << "Linked List: ";
displayList(head); // Display list
return 0;
}
Explanation of C++ Code Steps:
- Define the Node Class: Instead of a struct, we define a
Node
class withdata
andnext
. - Constructor: The
Node
constructor initializesdata
andnext
. - Insert at Beginning: Similar to C, this function creates a new node, links it to the head, and updates the head.
- Display List: Traverses and displays data for each node.
- Main Function: Inserts nodes and prints the linked list.
Code:
using System;
class Node {
public int data;
public Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
class LinkedList {
private Node head;
public void InsertAtBeginning(int newData) {
Node newNode = new Node(newData); // Create a new node
newNode.next = head; // Point new node to current head
head = newNode; // Update head to new node
}
public void DisplayList() {
Node node = head;
while (node != null) {
Console.Write(node.data + " ");
node = node.next;
}
}
static void Main(string[] args) {
LinkedList list = new LinkedList();
list.InsertAtBeginning(1); // Insert node with data 1
list.InsertAtBeginning(2); // Insert node with data 2
list.InsertAtBeginning(3); // Insert node with data 3
Console.Write("Linked List: ");
list.DisplayList(); // Display list
}
}
Explanation of C# Code Steps:
- Define the Node Class:
Node
class hasdata
andnext
. - Constructor: Initializes
data
andnext
tonull
. - Insert at Beginning: Adds a new node at the start by pointing it to the current head.
- Display List: Prints each node’s data.
- Main Function: Inserts nodes and displays the list.
Code:
class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
class LinkedList {
private Node head;
public void insertAtBeginning(int newData) {
Node newNode = new Node(newData); // Create new node
newNode.next = head; // Point new node to head
head = newNode; // Update head to new node
}
public void displayList() {
Node node = head;
while (node != null) {
System.out.print(node.data + " ");
node = node.next;
}
}
public static void main(String[] args) {
LinkedList list = new LinkedList();
list.insertAtBeginning(1); // Insert node with data 1
list.insertAtBeginning(2); // Insert node with data 2
list.insertAtBeginning(3); // Insert node with data 3
System.out.print("Linked List: ");
list.displayList(); // Display list
}
}
Explanation of Java Code Steps:
- Define Node Class: Java’s
Node
class containsdata
andnext
. - Constructor: Initializes the node’s data and sets
next
tonull
. - Insert at Beginning: Adds new node at start by updating the head.
- Display List: Traverses and prints each node’s data.
- Main Method: Creates a linked list, inserts nodes, and displays the list.
Code:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def insert_at_beginning(self, new_data):
new_node = Node(new_data) # Create new node
new_node.next = self.head # Link to head
self.head = new_node # Update head
def display_list(self):
current = self.head
while current:
print(current.data, end=" ")
current = current.next
# Driver code
if __name__ == "__main__":
ll = LinkedList()
ll.insert_at_beginning(1) # Insert node with data 1
ll.insert_at_beginning(2) # Insert node with data 2
ll.insert_at_beginning(3) # Insert node with data 3
print("Linked List:", end=" ")
ll.display_list() # Display list
Explanation of Python Code Steps:
- Define Node Class: The
Node
class holdsdata
andnext
. - Initialize LinkedList: Sets the head to
None
. - Insert at Beginning: Creates a new node, points it to the head, and updates the head.
- Display List: Traverses each node, printing
data
. - Driver Code: Inserts nodes into the list and displays it.
Output:
Linked List: 3 2 1
Summary of Differences Across Languages
- Memory Management: Languages like C and C++ require explicit memory management with
malloc
andnew
, while Java and Python use automatic memory management. - Pointers: C and C++ use pointers explicitly, while Java and C# use references. Python manages references implicitly.
- Syntax Differences: Each language has its own syntax for defining classes, structs, and functions. However, the logic remains consistent across implementations.
Each example illustrates the common data structure operations in a linked list with step-by-step explanations for better comprehension.
Conclusion
Linked lists are a versatile, dynamic data structure that provides a solution to many limitations associated with arrays. With their diverse types—singly linked lists, doubly linked lists, circular singly linked lists, and circular doubly linked lists—they offer flexibility in applications ranging from dynamic memory allocation to implementing complex data structures like stacks, queues, and graphs. While they come with some limitations, such as higher memory usage and slower traversal times, their benefits in dynamic environments make them indispensable in computer science.
Frequently Asked Questions (FAQs)
What is a Linked List?
A linked list is a linear data structure consisting of a series of connected nodes. Each node contains two parts: data (the value stored in the node) and a pointer (the address of the next node in the list). Unlike arrays, linked lists do not require a contiguous block of memory. Instead, each node can be stored anywhere in memory, linked together using pointers.
What are the types of Linked Lists?
There are four main types of linked lists:
- Singly Linked List: Each node points only to the next node in the list.
- Doubly Linked List: Each node has two pointers, one pointing to the next node and one to the previous node.
- Circular Singly Linked List: The last node’s pointer points back to the first node, forming a circular structure.
- Circular Doubly Linked List: Similar to a doubly linked list, but the last node points back to the first node, and the first node points back to the last node.
Why would we use Linked Lists over Arrays?
Linked lists offer several advantages over arrays:
- Dynamic Size: The size of a linked list can grow or shrink as needed, whereas an array has a fixed size.
- Efficient Insertion and Deletion: Adding or removing elements in a linked list is easier, as it only involves adjusting pointers without shifting elements.
- Memory Efficiency: Memory is allocated dynamically, making linked lists more flexible in terms of space usage.
However, arrays are generally faster for random access because linked lists require traversal from the head node to access an element.
What are the main disadvantages of using Linked Lists?
The primary disadvantages of linked lists are:
- Memory Usage: Each node in a linked list uses extra memory for pointers.
- Traversal Time: Accessing an element in a linked list requires traversing from the head node, which can be time-consuming.
- Reverse Traversing: Reverse traversal is complex in singly linked lists and requires extra memory (back pointers) in doubly linked lists.
How is memory managed in a Linked List?
In languages like C and C++, dynamic memory allocation functions such as malloc
or new
are used to allocate memory for new nodes. When a node is deleted, memory is freed using free
or delete
. In languages with automatic garbage collection (e.g., Java and Python), memory is managed by the runtime environment, which automatically frees memory when nodes are no longer in use.
What is a Node in a Linked List?
A node in a linked list is a basic building block that contains data and a pointer to the next node. In C, a node is typically defined as a structure:
struct Node {
int data; // Data part
struct Node *next; // Pointer to the next node
};
In Java, a node might be represented as a class:
class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
How do we insert a new node in a Linked List?
Inserting a new node can be done at different positions:
- At the Beginning: The new node is linked to the head node, and the head pointer is updated to point to the new node.
- At the End: We traverse to the last node and set its
next
pointer to the new node. - At a Specific Position: We locate the desired position, adjust the pointers of adjacent nodes, and insert the new node.
For example, in C, inserting at the beginning might look like this:
void insertAtBeginning(struct Node** head, int newData) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = newData;
newNode->next = *head;
*head = newNode;
}
What is the difference between a Singly and a Doubly Linked List?
- Singly Linked List: Each node has a single pointer pointing to the next node. Traversal is possible only in a forward direction.
- Doubly Linked List: Each node contains two pointers, one pointing to the next node and another pointing to the previous node, enabling both forward and backward traversal.
Singly-linked lists require less memory but limit traversal to one direction. Doubly linked lists allow bidirectional traversal but require additional memory to store the second pointer.
How do Linked Lists support dynamic memory allocation?
In linked lists, memory for each node is allocated dynamically when needed, allowing the list to grow and shrink as required. For instance, in C, malloc
is used to allocate memory during runtime, and free
is used to release memory. This dynamic allocation is especially useful for data structures with unpredictable or changing sizes.
In Python and Java, where automatic garbage collection is available, memory for unreferenced nodes is reclaimed by the garbage collector, removing the need for explicit memory management.
How can we implement other data structures using Linked Lists?
Linked lists are versatile and can be used to implement:
- Stacks: A stack can be implemented by restricting operations to the top of the linked list.
- Queues: A queue can be implemented by enqueuing at the end and dequeuing from the beginning of the list.
- Graphs: Adjacency lists are often implemented using linked lists to represent connections between vertices.
- Trees: Binary trees can use nodes with multiple pointers (e.g., left and right children) to form a tree structure.
For example, implementing a stack using a linked list involves inserting nodes at the beginning (push) and deleting nodes from the beginning (pop), as shown here in C:
void push(struct Node** top, int newData) {
insertAtBeginning(top, newData); // Use insert function
}
void pop(struct Node** top) {
if (*top == NULL) return;
struct Node* temp = *top;
*top = (*top)->next;
free(temp); // Free memory of removed node
}
In Python, a stack can be implemented similarly using a linked list as follows:
class Stack:
def __init__(self):
self.head = None
def push(self, data):
new_node = Node(data)
new_node.next = self.head
self.head = new_node
def pop(self):
if self.head is None:
return None
data = self.head.data
self.head = self.head.next
return data
Using linked lists in this way makes data structures flexible, allowing dynamic size changes and efficient insertions and deletions.