In programming, 2D arrays are a versatile and powerful data structure, often used to create table-like structures and implement relational database formats. A 2D array is essentially an array of arrays, organized as rows and columns. This matrix-like structure allows us to handle large datasets efficiently and simplifies data access, storage, and manipulation. In this article, we’ll explore how to declare, access, and initialize 2D arrays, along with their advantages and use cases. We’ll also provide multiple examples, and code to demonstrate each concept.
Table of Contents
What is a 2D Array?
A 2D array is a collection of elements arranged in a matrix format with rows and columns. Each element in the 2D array is identified by two indices, one for the row and one for the column. 2D arrays are commonly used to represent grids, tables, and other data sets that involve both horizontal and vertical relationships. For instance, spreadsheets, game boards, and pixel data in images are examples of data that can be represented by a 2D array.
In essence, 2D arrays provide a convenient way to manage complex datasets as a single variable, making it easier to pass large amounts of data to functions or algorithms.
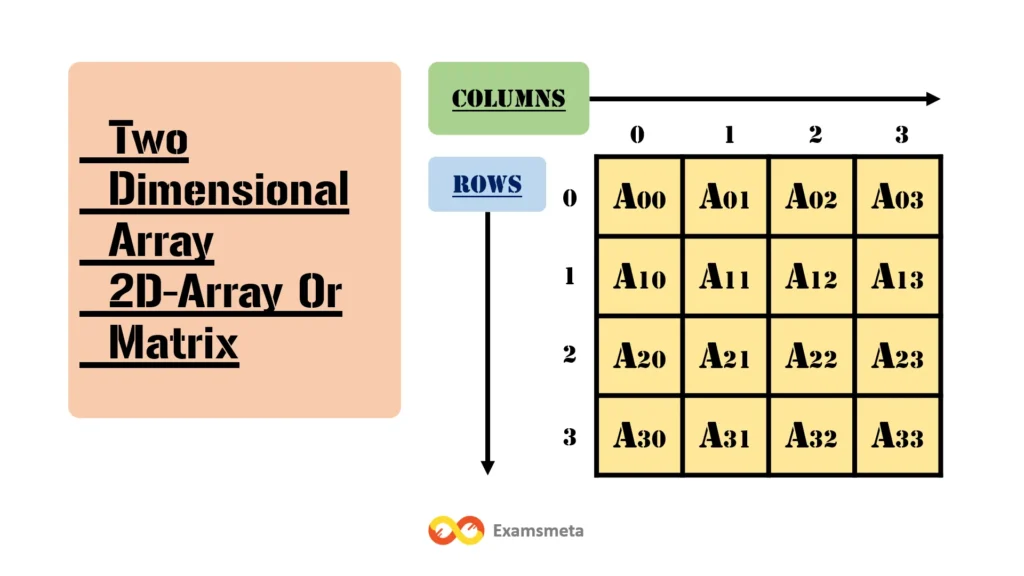
Declaring a 2D Array
The syntax to declare a 2D array is similar to a 1D array, with the addition of a second dimension:
int arr[max_rows][max_columns];
Here:
int
represents the data type of the elements (in this case, integers).max_rows
is the number of rows in the array.max_columns
is the number of columns in the array.
For example, to declare a 3×3 2D array to store integers:
int matrix[3][3];
This code defines a matrix with 3 rows and 3 columns, providing 9 cells in total for integer storage.
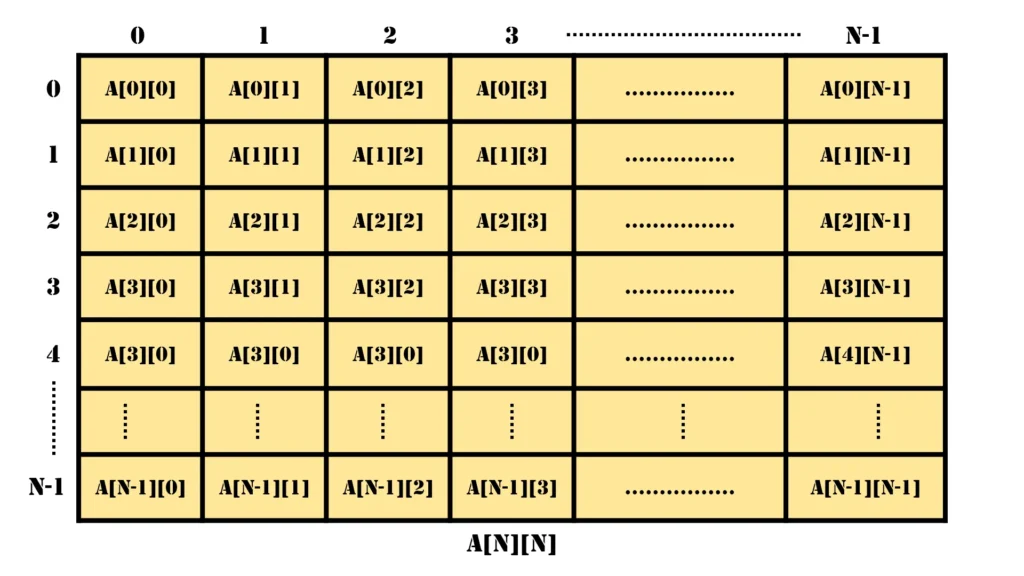
In the above image, a two-dimensional array is presented, where elements are structured in a grid format comprising rows and columns. Each element’s position in this array is specified by two indices, with the first index denoting the row and the second representing the column. For instance, the first element in the first row is identified as a[0][0], where “0” in the first index indicates it belongs to the first row, and “0” in the second index signifies it is in the first column.
This systematic arrangement allows for precise referencing and manipulation of data, as each cell in the array can be accessed based on its unique row and column indices.
This format is essential for various operations in programming, where structured data is managed efficiently by navigating through specified row and column coordinates, enabling complex computations and organization in a highly accessible form.
Accessing Data in a 2D Array
Data in a 2D array can be accessed using the indices of its rows and columns. Accessing a specific cell in a 2D array requires the row and column indices, just as we use a single index for accessing elements in a 1D array.
int x = matrix[i][j];
In this case:
matrix
is the 2D array.i
andj
are the indices for the row and column, respectively.- The value at position
(i, j)
is stored in the variablex
.
For example, if you wanted to access the element in the first row and second column, you would use:
int value = matrix[0][1];
Note: In programming, array indices generally start from 0, so the first element is matrix[0][0]
.
Setting Values in a 2D Array
You can assign values to individual elements in a 2D array. Suppose you want to set each cell of a 3×3 array to 0. A nested for
loop is commonly used for this purpose:
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
matrix[i][j] = 0;
}
}
The outer loop iterates over each row, while the inner loop iterates over each column, setting every element to 0.
Initializing 2D Arrays
In many programming languages, we can initialize a 1D array without specifying its size. However, with 2D arrays, at least the second dimension must be specified during initialization. This allows the compiler to allocate memory properly.
The syntax to initialize a 2D array with values is as follows:
int arr[2][2] = {{0, 1}, {2, 3}};
Here:
arr
is a 2×2 2D array initialized with values0, 1, 2,
and3
.- Each row is enclosed in braces
{}
.
This initialization represents the following structure in memory:
0 1
2 3
Automatic Size Calculation
If we omit the row count, the compiler can determine it based on the number of values provided. For example:
int arr[3][2] = {{0, 1}, {2, 3}, {4, 5}};
The compiler interprets this as a 3×2 array based on the given data.
Practical Applications of 2D Arrays
- Matrices: Used in mathematics and physics to represent grids or transformations.
- Image Processing: Representing pixels in rows and columns, where each pixel can be stored as an element in a 2D array.
- Game Boards: 2D arrays can represent boards for games like chess or tic-tac-toe.
- Tables and Spreadsheets: Organizing tabular data for easy access and manipulation.
Example Code: 2D Array Operations
Below is a complete example in C++ to demonstrate declaring, initializing, and accessing elements in a 2D array.
#include <iostream>
using namespace std;
int main() {
// Declare and initialize a 3x3 matrix
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
// Display elements of the matrix
cout << "Matrix elements:" << endl;
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << matrix[i][j] << " ";
}
cout << endl;
}
// Setting all elements to zero
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
matrix[i][j] = 0;
}
}
// Display the updated matrix
cout << "Matrix after setting all elements to 0:" << endl;
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << matrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
This program performs the following:
- Initializes a 3×3 matrix with numbers 1 through 9.
- Displays the matrix.
- Sets all elements to zero using nested loops.
- Displays the matrix after resetting values.
Output:
Matrix elements:
1 2 3
4 5 6
7 8 9
Matrix after setting all elements to 0:
0 0 0
0 0 0
0 0 0
Example Code: Multiple Programming Languages
Code:
The program is a 2D array input-output program that:
- Takes a 3×3 matrix input from the user.
- Displays the matrix after input.
Let’s break down each part of the C code and see how it works:
#include <stdio.h>
void main ()
{
int arr[3][3], i, j; // Declare a 3x3 integer array and two loop counters i and j
// First nested loop: Getting user input for each cell
for (i = 0; i < 3; i++)
{
for (j = 0; j < 3; j++)
{
printf("Enter a[%d][%d]: ", i, j); // Prompt user for input at each index
scanf("%d", &arr[i][j]); // Store input in arr[i][j]
}
}
// Second nested loop: Printing each cell's value
printf("\nPrinting the elements ...\n");
for (i = 0; i < 3; i++)
{
printf("\n"); // Newline for each row
for (j = 0; j < 3; j++)
{
printf("%d\t", arr[i][j]); // Print each element in row with tab separation
}
}
}
Explanation of Code in C
- Array Declaration:
int arr[3][3]
defines a 2D array with 3 rows and 3 columns. - Input Loop: The nested loop structure allows us to iterate through each cell of the 2D array, asking the user to provide a value for each cell.
- Output Loop: A second nested loop structure prints the array in matrix format.
Code:
In C++, we use similar I/O functions but employ iostream
for output.
#include <iostream>
using namespace std;
int main()
{
int arr[3][3]; // Declare a 3x3 array
// Input Loop
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
cout << "Enter a[" << i << "][" << j << "]: "; // Prompt
cin >> arr[i][j]; // Store value
}
}
// Output Loop
cout << "\nPrinting the elements ...\n";
for (int i = 0; i < 3; i++)
{
cout << endl;
for (int j = 0; j < 3; j++)
{
cout << arr[i][j] << "\t"; // Print each element
}
}
return 0;
}
Explanation of Code in C++
- I/O Functions:
cin
andcout
replacescanf
andprintf
. - Namespace:
using namespace std;
allows for easier access to standard library components.
Code:
In C#, we use the Console class for input/output. Arrays in C# are more flexible with properties such as .Length
.
using System;
class Program
{
static void Main()
{
int[,] arr = new int[3, 3]; // Declare a 2D array in C#
// Input Loop
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
Console.Write("Enter a[{0}][{1}]: ", i, j); // Prompt user
arr[i, j] = int.Parse(Console.ReadLine()); // Parse and store input
}
}
// Output Loop
Console.WriteLine("\nPrinting the elements ...");
for (int i = 0; i < 3; i++)
{
Console.WriteLine(); // Newline for each row
for (int j = 0; j < 3; j++)
{
Console.Write(arr[i, j] + "\t"); // Print each element
}
}
}
}
Explanation of Code in C#
- Input Parsing:
Console.ReadLine()
reads input as a string, soint.Parse()
converts it to an integer. - 2D Array Syntax:
[ , ]
syntax defines a 2D array in C#.
Code:
In Java, we use the Scanner class for input and System.out.print
for output.
import java.util.Scanner;
public class MatrixInputOutput
{
public static void main(String[] args)
{
int[][] arr = new int[3][3]; // Declare 3x3 array
Scanner scanner = new Scanner(System.in);
// Input Loop
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
System.out.print("Enter a[" + i + "][" + j + "]: "); // Prompt
arr[i][j] = scanner.nextInt(); // Store value
}
}
// Output Loop
System.out.println("\nPrinting the elements ...");
for (int i = 0; i < 3; i++)
{
System.out.println();
for (int j = 0; j < 3; j++)
{
System.out.print(arr[i][j] + "\t"); // Print each element
}
}
scanner.close();
}
}
Explanation of Code in Java
- Scanner Class:
Scanner scanner = new Scanner(System.in);
creates an object to take input. - Array Initialization: The syntax
int[][] arr = new int[3][3];
initializes a 3×3 array in Java.
Code:
In Python, arrays can be represented with lists of lists.
# 3x3 Matrix as List of Lists
arr = [[0] * 3 for _ in range(3)]
# Input Loop
for i in range(3):
for j in range(3):
arr[i][j] = int(input(f"Enter a[{i}][{j}]: "))
# Output Loop
print("\nPrinting the elements ...")
for i in range(3):
print()
for j in range(3):
print(arr[i][j], end="\t")
Explanation of Code in Python
- List Comprehension:
arr = [[0] * 3 for _ in range(3)]
initializes a 3×3 matrix. - Input and Print Functions:
input()
reads input, andprint()
displays output in Python.
Summary
Each language has unique syntax and features, but the general logic remains the same:
- Declare a 2D array (or equivalent).
- Loop to collect inputs for each cell.
- Loop to output the array in matrix format.
Output (Common to All Languages)
The output for all languages will be in the following format:
Enter a[0][0]: 1
Enter a[0][1]: 2
Enter a[0][2]: 3
Enter a[1][0]: 4
Enter a[1][1]: 5
Enter a[1][2]: 6
Enter a[2][0]: 7
Enter a[2][1]: 8
Enter a[2][2]: 9
Printing the elements ...
1 2 3
4 5 6
7 8 9
Each version of the program has the same logic but is adapted to the syntax and features of each programming language.
Conclusion
2D arrays are an essential tool in programming, providing a structured way to handle complex datasets. By arranging data in rows and columns, 2D arrays enable easy access and manipulation of data. From image processing to game development, they offer a powerful solution for representing and managing multi-dimensional data.
Understanding 2D arrays is foundational for working with more complex data structures. Familiarity with 2D arrays opens up possibilities for creating sophisticated algorithms, handling large datasets, and building data-intensive applications.
Frequently Asked Questions (FAQs) on 2D Arrays
What is a 2D Array?
A 2D array is a type of data structure that consists of a collection of elements arranged in rows and columns. Think of it as an array of arrays, or a matrix-like structure, where each element is accessible by two indices: one for the row and one for the column. This structure is particularly useful in representing grids, tables, and matrices, making it a go-to choice for data-heavy applications.
How do you declare a 2D array?
In most programming languages like C or C++, a 2D array is declared using the following syntax:
int array_name[rows][columns];
For example, to declare a 3×3 2D array of integers:
int matrix[3][3];
Here:
int
is the data type.matrix
is the name of the array.[3][3]
specifies that it has 3 rows and 3 columns.
How do you access elements in a 2D array?
Elements in a 2D array are accessed using two indices, one for the row and one for the column:
int element = array[row][column];
For example, if matrix
is a 2D array with 3 rows and 3 columns, matrix[0][1]
will access the element in the first row and second column. In programming, indices typically start from 0, so matrix[0][0]
represents the top-left element.
How do you initialize a 2D array with specific values?
To initialize a 2D array with values, you can use nested braces:
int matrix[2][2] = {{1, 2}, {3, 4}};
This creates a 2×2 matrix with the following structure:
1 2
3 4
If the row count is omitted, the compiler can infer it from the data provided.
Can you resize a 2D array after it is declared?
In languages like C or C++, arrays are static, meaning you cannot resize them after declaration. However, in languages like Python, which supports dynamic data structures, you can modify lists to behave like resizable 2D arrays.
To achieve dynamic resizing in C++, you could use vectors, a resizable array type in the Standard Template Library (STL).
What are the use cases of 2D arrays?
2D arrays are used in:
- Matrices for mathematical computations.
- Image processing, where each pixel can be represented by a matrix element.
- Game boards like chess or tic-tac-toe.
- Tables and spreadsheets, where data is stored in rows and columns.
- Graph adjacency matrices in graph theory.
How can you set all values of a 2D array to zero?
To initialize each cell of a 2D array to 0, you can use a nested for-loop:
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
array[i][j] = 0;
}
}
This loop iterates over each row and column, setting each element to zero.
Can you create a 2D array of different data types in each cell?
No, in most programming languages, all elements within an array must be of the same data type. However, you could use a 2D array of structures (structs) or a 2D array of objects in C++ and Java, respectively, to store complex data that includes multiple types.
How do 2D arrays differ from 1D arrays?
While 1D arrays store elements linearly, 2D arrays store elements in a matrix format with rows and columns. In 2D arrays, you need two indices (row and column) to access each element, unlike 1D arrays, which require only one index.
How is memory allocated for a 2D array?
In languages like C/C++, 2D arrays are often stored in row-major order. This means that elements in each row are stored in contiguous memory locations. For instance, if you declare int matrix[3][3]
, the memory will first store all elements of row 0, then row 1, and finally row 2. This layout allows for efficient memory access.
What is the maximum size of a 2D array?
The maximum size of a 2D array depends on the programming language and the system’s memory limit. For example, in C++, you can create very large arrays if there is enough heap memory available, but the maximum size might be limited by constraints such as stack size and compiler limits.
Can you pass a 2D array to a function?
Yes, 2D arrays can be passed to functions. In C++, you must specify the number of columns in the function’s parameter list, even if the number of rows is left unspecified. For example:
void processArray(int array[][3], int rows);
Alternatively, pointers can be used to pass 2D arrays to functions more flexibly.
How does initializing a 2D array with all elements affect performance?
Initializing a 2D array with all elements (such as setting all cells to zero) may consume time, especially for large arrays. The time complexity of such an operation is O(rows * columns)
, as each cell must be individually accessed and assigned.
What are some advantages of using 2D arrays?
2D arrays offer several benefits:
- Organized storage for complex datasets.
- Efficient data access with row and column indexing.
- Easy data manipulation for applications like image processing and grid-based simulations.
- Compatibility with algorithms that rely on matrix operations.
What are the limitations of 2D arrays?
Some limitations of 2D arrays include:
- Fixed-size in languages without dynamic array support.
- Memory constraints for large arrays, especially if stored on the stack.
- Restricted data type consistency within each array.
In some cases, linked lists, dictionaries, or hashmaps might be preferable for applications needing flexible or sparse structures.